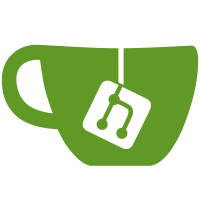
Other than being a bit nicer than passing function pointers all over the place, this helps dxgi/d3d10. While the swapchain itself is created in dxgi, its surfaces are constructed in d3d10core, which makes it impractical for dxgi to pass the appropriate function pointers.
3444 lines
133 KiB
Plaintext
3444 lines
133 KiB
Plaintext
/*
|
|
* Copyright 2002-2003 The wine-d3d team
|
|
* Copyright 2002-2003 Jason Edmeades
|
|
* Copyright 2002-2003 Raphael Junqueira
|
|
* Copyright 2005 Oliver Stieber
|
|
* Copyright 2006 Stefan Dösinger
|
|
* Copyright 2006 Stefan Dösinger for CodeWeavers
|
|
* Copyright 2007 Henri Verbeet
|
|
* Copyright 2008 Henri Verbeet for CodeWeavers
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301, USA
|
|
*/
|
|
|
|
import "unknwn.idl";
|
|
|
|
cpp_quote("#if 0")
|
|
typedef HANDLE HMONITOR;
|
|
|
|
typedef struct _RGNDATAHEADER
|
|
{
|
|
DWORD dwSize;
|
|
DWORD iType;
|
|
DWORD nCount;
|
|
DWORD nRgnSize;
|
|
RECT rcBound;
|
|
} RGNDATAHEADER;
|
|
|
|
typedef struct _RGNDATA
|
|
{
|
|
RGNDATAHEADER rdh;
|
|
char Buffer[1];
|
|
} RGNDATA;
|
|
cpp_quote("#endif")
|
|
|
|
cpp_quote("#define WINED3D_OK S_OK")
|
|
|
|
const UINT _FACWINED3D = 0x876;
|
|
cpp_quote("#define MAKE_WINED3DSTATUS(code) MAKE_HRESULT(0, _FACWINED3D, code)")
|
|
cpp_quote("#define WINED3DOK_NOAUTOGEN MAKE_WINED3DSTATUS(2159)")
|
|
|
|
cpp_quote("#define MAKE_WINED3DHRESULT(code) MAKE_HRESULT(1, _FACWINED3D, code)")
|
|
cpp_quote("#define WINED3DERR_WRONGTEXTUREFORMAT MAKE_WINED3DHRESULT(2072)")
|
|
cpp_quote("#define WINED3DERR_UNSUPPORTEDCOLOROPERATION MAKE_WINED3DHRESULT(2073)")
|
|
cpp_quote("#define WINED3DERR_UNSUPPORTEDCOLORARG MAKE_WINED3DHRESULT(2074)")
|
|
cpp_quote("#define WINED3DERR_UNSUPPORTEDALPHAOPERATION MAKE_WINED3DHRESULT(2075)")
|
|
cpp_quote("#define WINED3DERR_UNSUPPORTEDALPHAARG MAKE_WINED3DHRESULT(2076)")
|
|
cpp_quote("#define WINED3DERR_TOOMANYOPERATIONS MAKE_WINED3DHRESULT(2077)")
|
|
cpp_quote("#define WINED3DERR_CONFLICTINGTEXTUREFILTER MAKE_WINED3DHRESULT(2078)")
|
|
cpp_quote("#define WINED3DERR_UNSUPPORTEDFACTORVALUE MAKE_WINED3DHRESULT(2079)")
|
|
cpp_quote("#define WINED3DERR_CONFLICTINGRENDERSTATE MAKE_WINED3DHRESULT(2081)")
|
|
cpp_quote("#define WINED3DERR_UNSUPPORTEDTEXTUREFILTER MAKE_WINED3DHRESULT(2082)")
|
|
cpp_quote("#define WINED3DERR_CONFLICTINGTEXTUREPALETTE MAKE_WINED3DHRESULT(2086)")
|
|
cpp_quote("#define WINED3DERR_DRIVERINTERNALERROR MAKE_WINED3DHRESULT(2087)")
|
|
cpp_quote("#define WINED3DERR_NOTFOUND MAKE_WINED3DHRESULT(2150)")
|
|
cpp_quote("#define WINED3DERR_MOREDATA MAKE_WINED3DHRESULT(2151)")
|
|
cpp_quote("#define WINED3DERR_DEVICELOST MAKE_WINED3DHRESULT(2152)")
|
|
cpp_quote("#define WINED3DERR_DEVICENOTRESET MAKE_WINED3DHRESULT(2153)")
|
|
cpp_quote("#define WINED3DERR_NOTAVAILABLE MAKE_WINED3DHRESULT(2154)")
|
|
cpp_quote("#define WINED3DERR_OUTOFVIDEOMEMORY MAKE_WINED3DHRESULT(380)")
|
|
cpp_quote("#define WINED3DERR_INVALIDDEVICE MAKE_WINED3DHRESULT(2155)")
|
|
cpp_quote("#define WINED3DERR_INVALIDCALL MAKE_WINED3DHRESULT(2156)")
|
|
cpp_quote("#define WINED3DERR_DRIVERINVALIDCALL MAKE_WINED3DHRESULT(2157)")
|
|
cpp_quote("#define WINED3DERR_WASSTILLDRAWING MAKE_WINED3DHRESULT(540)")
|
|
cpp_quote("#define WINEDDERR_NOTAOVERLAYSURFACE MAKE_WINED3DHRESULT(580)")
|
|
cpp_quote("#define WINEDDERR_NOTLOCKED MAKE_WINED3DHRESULT(584)")
|
|
cpp_quote("#define WINEDDERR_NODC MAKE_WINED3DHRESULT(586)")
|
|
cpp_quote("#define WINEDDERR_DCALREADYCREATED MAKE_WINED3DHRESULT(620)")
|
|
cpp_quote("#define WINEDDERR_NOTFLIPPABLE MAKE_WINED3DHRESULT(582)")
|
|
cpp_quote("#define WINEDDERR_SURFACEBUSY MAKE_WINED3DHRESULT(430)")
|
|
cpp_quote("#define WINEDDERR_INVALIDRECT MAKE_WINED3DHRESULT(150)")
|
|
cpp_quote("#define WINEDDERR_NOCLIPLIST MAKE_WINED3DHRESULT(205)")
|
|
cpp_quote("#define WINEDDERR_OVERLAYNOTVISIBLE MAKE_WINED3DHRESULT(577)")
|
|
|
|
typedef DWORD WINED3DCOLOR;
|
|
|
|
typedef enum _WINED3DLIGHTTYPE
|
|
{
|
|
WINED3DLIGHT_POINT = 1,
|
|
WINED3DLIGHT_SPOT = 2,
|
|
WINED3DLIGHT_DIRECTIONAL = 3,
|
|
WINED3DLIGHT_PARALLELPOINT = 4, /* D3D7 */
|
|
WINED3DLIGHT_GLSPOT = 5, /* D3D7 */
|
|
WINED3DLIGHT_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DLIGHTTYPE;
|
|
|
|
typedef enum _WINED3DPRIMITIVETYPE
|
|
{
|
|
WINED3DPT_POINTLIST = 1,
|
|
WINED3DPT_LINELIST = 2,
|
|
WINED3DPT_LINESTRIP = 3,
|
|
WINED3DPT_TRIANGLELIST = 4,
|
|
WINED3DPT_TRIANGLESTRIP = 5,
|
|
WINED3DPT_TRIANGLEFAN = 6,
|
|
WINED3DPT_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DPRIMITIVETYPE;
|
|
|
|
typedef enum _WINED3DDEVTYPE
|
|
{
|
|
WINED3DDEVTYPE_HAL = 1,
|
|
WINED3DDEVTYPE_REF = 2,
|
|
WINED3DDEVTYPE_SW = 3,
|
|
WINED3DDEVTYPE_NULLREF = 4,
|
|
WINED3DDEVTYPE_FORCE_DWORD = 0xffffffff
|
|
} WINED3DDEVTYPE;
|
|
|
|
typedef enum _WINED3DDEGREETYPE
|
|
{
|
|
WINED3DDEGREE_LINEAR = 1,
|
|
WINED3DDEGREE_QUADRATIC = 2,
|
|
WINED3DDEGREE_CUBIC = 3,
|
|
WINED3DDEGREE_QUINTIC = 5,
|
|
WINED3DDEGREE_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DDEGREETYPE;
|
|
|
|
typedef enum _WINED3DFORMAT
|
|
{
|
|
WINED3DFMT_UNKNOWN = 0,
|
|
WINED3DFMT_R8G8B8 = 20,
|
|
WINED3DFMT_A8R8G8B8 = 21,
|
|
WINED3DFMT_X8R8G8B8 = 22,
|
|
WINED3DFMT_R5G6B5 = 23,
|
|
WINED3DFMT_X1R5G5B5 = 24,
|
|
WINED3DFMT_A1R5G5B5 = 25,
|
|
WINED3DFMT_A4R4G4B4 = 26,
|
|
WINED3DFMT_R3G3B2 = 27,
|
|
WINED3DFMT_A8 = 28,
|
|
WINED3DFMT_A8R3G3B2 = 29,
|
|
WINED3DFMT_X4R4G4B4 = 30,
|
|
WINED3DFMT_A2B10G10R10 = 31,
|
|
WINED3DFMT_A8B8G8R8 = 32,
|
|
WINED3DFMT_X8B8G8R8 = 33,
|
|
WINED3DFMT_G16R16 = 34,
|
|
WINED3DFMT_A2R10G10B10 = 35,
|
|
WINED3DFMT_A16B16G16R16 = 36,
|
|
WINED3DFMT_A8P8 = 40,
|
|
WINED3DFMT_P8 = 41,
|
|
WINED3DFMT_L8 = 50,
|
|
WINED3DFMT_A8L8 = 51,
|
|
WINED3DFMT_A4L4 = 52,
|
|
WINED3DFMT_V8U8 = 60,
|
|
WINED3DFMT_L6V5U5 = 61,
|
|
WINED3DFMT_X8L8V8U8 = 62,
|
|
WINED3DFMT_Q8W8V8U8 = 63,
|
|
WINED3DFMT_V16U16 = 64,
|
|
WINED3DFMT_W11V11U10 = 65,
|
|
WINED3DFMT_A2W10V10U10 = 67,
|
|
WINED3DFMT_D16_LOCKABLE = 70,
|
|
WINED3DFMT_D32 = 71,
|
|
WINED3DFMT_D15S1 = 73,
|
|
WINED3DFMT_D24S8 = 75,
|
|
WINED3DFMT_D24X8 = 77,
|
|
WINED3DFMT_D24X4S4 = 79,
|
|
WINED3DFMT_D16 = 80,
|
|
WINED3DFMT_L16 = 81,
|
|
WINED3DFMT_D32F_LOCKABLE = 82,
|
|
WINED3DFMT_D24FS8 = 83,
|
|
WINED3DFMT_VERTEXDATA = 100,
|
|
WINED3DFMT_INDEX16 = 101,
|
|
WINED3DFMT_INDEX32 = 102,
|
|
WINED3DFMT_Q16W16V16U16 = 110,
|
|
WINED3DFMT_R16F = 111,
|
|
WINED3DFMT_G16R16F = 112,
|
|
WINED3DFMT_A16B16G16R16F = 113,
|
|
WINED3DFMT_R32F = 114,
|
|
WINED3DFMT_G32R32F = 115,
|
|
WINED3DFMT_A32B32G32R32F = 116,
|
|
WINED3DFMT_CxV8U8 = 117,
|
|
WINED3DFMT_FORCE_DWORD = 0xffffffff
|
|
} WINED3DFORMAT;
|
|
cpp_quote("#define WINEMAKEFOURCC(ch0, ch1, ch2, ch3) \\")
|
|
cpp_quote(" ((DWORD)(BYTE)(ch0) | ((DWORD)(BYTE)(ch1) << 8) | \\")
|
|
cpp_quote(" ((DWORD)(BYTE)(ch2) << 16) | ((DWORD)(BYTE)(ch3) << 24 ))")
|
|
cpp_quote("#define WINED3DFMT_UYVY WINEMAKEFOURCC('U', 'Y', 'V', 'Y')")
|
|
cpp_quote("#define WINED3DFMT_YUY2 WINEMAKEFOURCC('Y', 'U', 'Y', '2')")
|
|
cpp_quote("#define WINED3DFMT_YV12 WINEMAKEFOURCC('Y', 'V', '1', '2')")
|
|
cpp_quote("#define WINED3DFMT_DXT1 WINEMAKEFOURCC('D', 'X', 'T', '1')")
|
|
cpp_quote("#define WINED3DFMT_DXT2 WINEMAKEFOURCC('D', 'X', 'T', '2')")
|
|
cpp_quote("#define WINED3DFMT_DXT3 WINEMAKEFOURCC('D', 'X', 'T', '3')")
|
|
cpp_quote("#define WINED3DFMT_DXT4 WINEMAKEFOURCC('D', 'X', 'T', '4')")
|
|
cpp_quote("#define WINED3DFMT_DXT5 WINEMAKEFOURCC('D', 'X', 'T', '5')")
|
|
cpp_quote("#define WINED3DFMT_MULTI2_ARGB8 WINEMAKEFOURCC('M', 'E', 'T', '1')")
|
|
cpp_quote("#define WINED3DFMT_G8R8_G8B8 WINEMAKEFOURCC('G', 'R', 'G', 'B')")
|
|
cpp_quote("#define WINED3DFMT_R8G8_B8G8 WINEMAKEFOURCC('R', 'G', 'B', 'G')")
|
|
/* Vendor specific formats */
|
|
cpp_quote("#define WINED3DFMT_ATI2N WINEMAKEFOURCC('A', 'T', 'I', '2')")
|
|
cpp_quote("#define WINED3DFMT_NVHU WINEMAKEFOURCC('N', 'V', 'H', 'U')")
|
|
cpp_quote("#define WINED3DFMT_NVHS WINEMAKEFOURCC('N', 'V', 'H', 'S')")
|
|
|
|
typedef enum _WINED3DRENDERSTATETYPE
|
|
{
|
|
WINED3DRS_TEXTUREHANDLE = 1, /* d3d7 */
|
|
WINED3DRS_ANTIALIAS = 2, /* d3d7 */
|
|
WINED3DRS_TEXTUREADDRESS = 3, /* d3d7 */
|
|
WINED3DRS_TEXTUREPERSPECTIVE = 4, /* d3d7 */
|
|
WINED3DRS_WRAPU = 5, /* d3d7 */
|
|
WINED3DRS_WRAPV = 6, /* d3d7 */
|
|
WINED3DRS_ZENABLE = 7,
|
|
WINED3DRS_FILLMODE = 8,
|
|
WINED3DRS_SHADEMODE = 9,
|
|
WINED3DRS_LINEPATTERN = 10, /* d3d7, d3d8 */
|
|
WINED3DRS_MONOENABLE = 11, /* d3d7 */
|
|
WINED3DRS_ROP2 = 12, /* d3d7 */
|
|
WINED3DRS_PLANEMASK = 13, /* d3d7 */
|
|
WINED3DRS_ZWRITEENABLE = 14,
|
|
WINED3DRS_ALPHATESTENABLE = 15,
|
|
WINED3DRS_LASTPIXEL = 16,
|
|
WINED3DRS_TEXTUREMAG = 17, /* d3d7 */
|
|
WINED3DRS_TEXTUREMIN = 18, /* d3d7 */
|
|
WINED3DRS_SRCBLEND = 19,
|
|
WINED3DRS_DESTBLEND = 20,
|
|
WINED3DRS_TEXTUREMAPBLEND = 21, /* d3d7 */
|
|
WINED3DRS_CULLMODE = 22,
|
|
WINED3DRS_ZFUNC = 23,
|
|
WINED3DRS_ALPHAREF = 24,
|
|
WINED3DRS_ALPHAFUNC = 25,
|
|
WINED3DRS_DITHERENABLE = 26,
|
|
WINED3DRS_ALPHABLENDENABLE = 27,
|
|
WINED3DRS_FOGENABLE = 28,
|
|
WINED3DRS_SPECULARENABLE = 29,
|
|
WINED3DRS_ZVISIBLE = 30, /* d3d7, d3d8 */
|
|
WINED3DRS_SUBPIXEL = 31, /* d3d7 */
|
|
WINED3DRS_SUBPIXELX = 32, /* d3d7 */
|
|
WINED3DRS_STIPPLEDALPHA = 33, /* d3d7 */
|
|
WINED3DRS_FOGCOLOR = 34,
|
|
WINED3DRS_FOGTABLEMODE = 35,
|
|
WINED3DRS_FOGSTART = 36,
|
|
WINED3DRS_FOGEND = 37,
|
|
WINED3DRS_FOGDENSITY = 38,
|
|
WINED3DRS_STIPPLEENABLE = 39, /* d3d7 */
|
|
WINED3DRS_EDGEANTIALIAS = 40, /* d3d7, d3d8 */
|
|
WINED3DRS_COLORKEYENABLE = 41, /* d3d7 */
|
|
WINED3DRS_BORDERCOLOR = 43, /* d3d7 */
|
|
WINED3DRS_TEXTUREADDRESSU = 44, /* d3d7 */
|
|
WINED3DRS_TEXTUREADDRESSV = 45, /* d3d7 */
|
|
WINED3DRS_MIPMAPLODBIAS = 46, /* d3d7 */
|
|
WINED3DRS_ZBIAS = 47, /* d3d7, d3d8 */
|
|
WINED3DRS_RANGEFOGENABLE = 48,
|
|
WINED3DRS_ANISOTROPY = 49, /* d3d7 */
|
|
WINED3DRS_FLUSHBATCH = 50, /* d3d7 */
|
|
WINED3DRS_TRANSLUCENTSORTINDEPENDENT = 51, /* d3d7 */
|
|
WINED3DRS_STENCILENABLE = 52,
|
|
WINED3DRS_STENCILFAIL = 53,
|
|
WINED3DRS_STENCILZFAIL = 54,
|
|
WINED3DRS_STENCILPASS = 55,
|
|
WINED3DRS_STENCILFUNC = 56,
|
|
WINED3DRS_STENCILREF = 57,
|
|
WINED3DRS_STENCILMASK = 58,
|
|
WINED3DRS_STENCILWRITEMASK = 59,
|
|
WINED3DRS_TEXTUREFACTOR = 60,
|
|
WINED3DRS_STIPPLEPATTERN00 = 64,
|
|
WINED3DRS_STIPPLEPATTERN01 = 65,
|
|
WINED3DRS_STIPPLEPATTERN02 = 66,
|
|
WINED3DRS_STIPPLEPATTERN03 = 67,
|
|
WINED3DRS_STIPPLEPATTERN04 = 68,
|
|
WINED3DRS_STIPPLEPATTERN05 = 69,
|
|
WINED3DRS_STIPPLEPATTERN06 = 70,
|
|
WINED3DRS_STIPPLEPATTERN07 = 71,
|
|
WINED3DRS_STIPPLEPATTERN08 = 72,
|
|
WINED3DRS_STIPPLEPATTERN09 = 73,
|
|
WINED3DRS_STIPPLEPATTERN10 = 74,
|
|
WINED3DRS_STIPPLEPATTERN11 = 75,
|
|
WINED3DRS_STIPPLEPATTERN12 = 76,
|
|
WINED3DRS_STIPPLEPATTERN13 = 77,
|
|
WINED3DRS_STIPPLEPATTERN14 = 78,
|
|
WINED3DRS_STIPPLEPATTERN15 = 79,
|
|
WINED3DRS_STIPPLEPATTERN16 = 80,
|
|
WINED3DRS_STIPPLEPATTERN17 = 81,
|
|
WINED3DRS_STIPPLEPATTERN18 = 82,
|
|
WINED3DRS_STIPPLEPATTERN19 = 83,
|
|
WINED3DRS_STIPPLEPATTERN20 = 84,
|
|
WINED3DRS_STIPPLEPATTERN21 = 85,
|
|
WINED3DRS_STIPPLEPATTERN22 = 86,
|
|
WINED3DRS_STIPPLEPATTERN23 = 87,
|
|
WINED3DRS_STIPPLEPATTERN24 = 88,
|
|
WINED3DRS_STIPPLEPATTERN25 = 89,
|
|
WINED3DRS_STIPPLEPATTERN26 = 90,
|
|
WINED3DRS_STIPPLEPATTERN27 = 91,
|
|
WINED3DRS_STIPPLEPATTERN28 = 92,
|
|
WINED3DRS_STIPPLEPATTERN29 = 93,
|
|
WINED3DRS_STIPPLEPATTERN30 = 94,
|
|
WINED3DRS_STIPPLEPATTERN31 = 95,
|
|
WINED3DRS_WRAP0 = 128,
|
|
WINED3DRS_WRAP1 = 129,
|
|
WINED3DRS_WRAP2 = 130,
|
|
WINED3DRS_WRAP3 = 131,
|
|
WINED3DRS_WRAP4 = 132,
|
|
WINED3DRS_WRAP5 = 133,
|
|
WINED3DRS_WRAP6 = 134,
|
|
WINED3DRS_WRAP7 = 135,
|
|
WINED3DRS_CLIPPING = 136,
|
|
WINED3DRS_LIGHTING = 137,
|
|
WINED3DRS_EXTENTS = 138, /* d3d7 */
|
|
WINED3DRS_AMBIENT = 139,
|
|
WINED3DRS_FOGVERTEXMODE = 140,
|
|
WINED3DRS_COLORVERTEX = 141,
|
|
WINED3DRS_LOCALVIEWER = 142,
|
|
WINED3DRS_NORMALIZENORMALS = 143,
|
|
WINED3DRS_COLORKEYBLENDENABLE = 144, /* d3d7 */
|
|
WINED3DRS_DIFFUSEMATERIALSOURCE = 145,
|
|
WINED3DRS_SPECULARMATERIALSOURCE = 146,
|
|
WINED3DRS_AMBIENTMATERIALSOURCE = 147,
|
|
WINED3DRS_EMISSIVEMATERIALSOURCE = 148,
|
|
WINED3DRS_VERTEXBLEND = 151,
|
|
WINED3DRS_CLIPPLANEENABLE = 152,
|
|
WINED3DRS_SOFTWAREVERTEXPROCESSING = 153, /* d3d8 */
|
|
WINED3DRS_POINTSIZE = 154,
|
|
WINED3DRS_POINTSIZE_MIN = 155,
|
|
WINED3DRS_POINTSPRITEENABLE = 156,
|
|
WINED3DRS_POINTSCALEENABLE = 157,
|
|
WINED3DRS_POINTSCALE_A = 158,
|
|
WINED3DRS_POINTSCALE_B = 159,
|
|
WINED3DRS_POINTSCALE_C = 160,
|
|
WINED3DRS_MULTISAMPLEANTIALIAS = 161,
|
|
WINED3DRS_MULTISAMPLEMASK = 162,
|
|
WINED3DRS_PATCHEDGESTYLE = 163,
|
|
WINED3DRS_PATCHSEGMENTS = 164, /* d3d8 */
|
|
WINED3DRS_DEBUGMONITORTOKEN = 165,
|
|
WINED3DRS_POINTSIZE_MAX = 166,
|
|
WINED3DRS_INDEXEDVERTEXBLENDENABLE = 167,
|
|
WINED3DRS_COLORWRITEENABLE = 168,
|
|
WINED3DRS_TWEENFACTOR = 170,
|
|
WINED3DRS_BLENDOP = 171,
|
|
WINED3DRS_POSITIONORDER = 172,
|
|
WINED3DRS_NORMALORDER = 173,
|
|
WINED3DRS_POSITIONDEGREE = 172,
|
|
WINED3DRS_NORMALDEGREE = 173,
|
|
WINED3DRS_SCISSORTESTENABLE = 174,
|
|
WINED3DRS_SLOPESCALEDEPTHBIAS = 175,
|
|
WINED3DRS_ANTIALIASEDLINEENABLE = 176,
|
|
WINED3DRS_MINTESSELLATIONLEVEL = 178,
|
|
WINED3DRS_MAXTESSELLATIONLEVEL = 179,
|
|
WINED3DRS_ADAPTIVETESS_X = 180,
|
|
WINED3DRS_ADAPTIVETESS_Y = 181,
|
|
WINED3DRS_ADAPTIVETESS_Z = 182,
|
|
WINED3DRS_ADAPTIVETESS_W = 183,
|
|
WINED3DRS_ENABLEADAPTIVETESSELLATION = 184,
|
|
WINED3DRS_TWOSIDEDSTENCILMODE = 185,
|
|
WINED3DRS_CCW_STENCILFAIL = 186,
|
|
WINED3DRS_CCW_STENCILZFAIL = 187,
|
|
WINED3DRS_CCW_STENCILPASS = 188,
|
|
WINED3DRS_CCW_STENCILFUNC = 189,
|
|
WINED3DRS_COLORWRITEENABLE1 = 190,
|
|
WINED3DRS_COLORWRITEENABLE2 = 191,
|
|
WINED3DRS_COLORWRITEENABLE3 = 192,
|
|
WINED3DRS_BLENDFACTOR = 193,
|
|
WINED3DRS_SRGBWRITEENABLE = 194,
|
|
WINED3DRS_DEPTHBIAS = 195,
|
|
WINED3DRS_WRAP8 = 198,
|
|
WINED3DRS_WRAP9 = 199,
|
|
WINED3DRS_WRAP10 = 200,
|
|
WINED3DRS_WRAP11 = 201,
|
|
WINED3DRS_WRAP12 = 202,
|
|
WINED3DRS_WRAP13 = 203,
|
|
WINED3DRS_WRAP14 = 204,
|
|
WINED3DRS_WRAP15 = 205,
|
|
WINED3DRS_SEPARATEALPHABLENDENABLE = 206,
|
|
WINED3DRS_SRCBLENDALPHA = 207,
|
|
WINED3DRS_DESTBLENDALPHA = 208,
|
|
WINED3DRS_BLENDOPALPHA = 209,
|
|
WINED3DRS_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DRENDERSTATETYPE;
|
|
const UINT WINEHIGHEST_RENDER_STATE = WINED3DRS_BLENDOPALPHA;
|
|
|
|
typedef enum _WINED3DBLEND
|
|
{
|
|
WINED3DBLEND_ZERO = 1,
|
|
WINED3DBLEND_ONE = 2,
|
|
WINED3DBLEND_SRCCOLOR = 3,
|
|
WINED3DBLEND_INVSRCCOLOR = 4,
|
|
WINED3DBLEND_SRCALPHA = 5,
|
|
WINED3DBLEND_INVSRCALPHA = 6,
|
|
WINED3DBLEND_DESTALPHA = 7,
|
|
WINED3DBLEND_INVDESTALPHA = 8,
|
|
WINED3DBLEND_DESTCOLOR = 9,
|
|
WINED3DBLEND_INVDESTCOLOR = 10,
|
|
WINED3DBLEND_SRCALPHASAT = 11,
|
|
WINED3DBLEND_BOTHSRCALPHA = 12,
|
|
WINED3DBLEND_BOTHINVSRCALPHA = 13,
|
|
WINED3DBLEND_BLENDFACTOR = 14,
|
|
WINED3DBLEND_INVBLENDFACTOR = 15,
|
|
WINED3DBLEND_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DBLEND;
|
|
|
|
typedef enum _WINED3DBLENDOP
|
|
{
|
|
WINED3DBLENDOP_ADD = 1,
|
|
WINED3DBLENDOP_SUBTRACT = 2,
|
|
WINED3DBLENDOP_REVSUBTRACT = 3,
|
|
WINED3DBLENDOP_MIN = 4,
|
|
WINED3DBLENDOP_MAX = 5,
|
|
WINED3DBLENDOP_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DBLENDOP;
|
|
|
|
typedef enum _WINED3DVERTEXBLENDFLAGS
|
|
{
|
|
WINED3DVBF_DISABLE = 0,
|
|
WINED3DVBF_1WEIGHTS = 1,
|
|
WINED3DVBF_2WEIGHTS = 2,
|
|
WINED3DVBF_3WEIGHTS = 3,
|
|
WINED3DVBF_TWEENING = 255,
|
|
WINED3DVBF_0WEIGHTS = 256
|
|
} WINED3DVERTEXBLENDFLAGS;
|
|
|
|
typedef enum _WINED3DCMPFUNC
|
|
{
|
|
WINED3DCMP_NEVER = 1,
|
|
WINED3DCMP_LESS = 2,
|
|
WINED3DCMP_EQUAL = 3,
|
|
WINED3DCMP_LESSEQUAL = 4,
|
|
WINED3DCMP_GREATER = 5,
|
|
WINED3DCMP_NOTEQUAL = 6,
|
|
WINED3DCMP_GREATEREQUAL = 7,
|
|
WINED3DCMP_ALWAYS = 8,
|
|
WINED3DCMP_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DCMPFUNC;
|
|
|
|
typedef enum _WINED3DZBUFFERTYPE
|
|
{
|
|
WINED3DZB_FALSE = 0,
|
|
WINED3DZB_TRUE = 1,
|
|
WINED3DZB_USEW = 2,
|
|
WINED3DZB_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DZBUFFERTYPE;
|
|
|
|
typedef enum _WINED3DFOGMODE
|
|
{
|
|
WINED3DFOG_NONE = 0,
|
|
WINED3DFOG_EXP = 1,
|
|
WINED3DFOG_EXP2 = 2,
|
|
WINED3DFOG_LINEAR = 3,
|
|
WINED3DFOG_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DFOGMODE;
|
|
|
|
typedef enum _WINED3DSHADEMODE
|
|
{
|
|
WINED3DSHADE_FLAT = 1,
|
|
WINED3DSHADE_GOURAUD = 2,
|
|
WINED3DSHADE_PHONG = 3,
|
|
WINED3DSHADE_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DSHADEMODE;
|
|
|
|
typedef enum _WINED3DFILLMODE
|
|
{
|
|
WINED3DFILL_POINT = 1,
|
|
WINED3DFILL_WIREFRAME = 2,
|
|
WINED3DFILL_SOLID = 3,
|
|
WINED3DFILL_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DFILLMODE;
|
|
|
|
typedef enum _WINED3DCULL
|
|
{
|
|
WINED3DCULL_NONE = 1,
|
|
WINED3DCULL_CW = 2,
|
|
WINED3DCULL_CCW = 3,
|
|
WINED3DCULL_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DCULL;
|
|
|
|
typedef enum _WINED3DSTENCILOP
|
|
{
|
|
WINED3DSTENCILOP_KEEP = 1,
|
|
WINED3DSTENCILOP_ZERO = 2,
|
|
WINED3DSTENCILOP_REPLACE = 3,
|
|
WINED3DSTENCILOP_INCRSAT = 4,
|
|
WINED3DSTENCILOP_DECRSAT = 5,
|
|
WINED3DSTENCILOP_INVERT = 6,
|
|
WINED3DSTENCILOP_INCR = 7,
|
|
WINED3DSTENCILOP_DECR = 8,
|
|
WINED3DSTENCILOP_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DSTENCILOP;
|
|
|
|
typedef enum _WINED3DMATERIALCOLORSOURCE
|
|
{
|
|
WINED3DMCS_MATERIAL = 0,
|
|
WINED3DMCS_COLOR1 = 1,
|
|
WINED3DMCS_COLOR2 = 2,
|
|
WINED3DMCS_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DMATERIALCOLORSOURCE;
|
|
|
|
typedef enum _WINED3DPATCHEDGESTYLE
|
|
{
|
|
WINED3DPATCHEDGE_DISCRETE = 0,
|
|
WINED3DPATCHEDGE_CONTINUOUS = 1,
|
|
WINED3DPATCHEDGE_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DPATCHEDGESTYLE;
|
|
|
|
typedef enum _WINED3DBACKBUFFER_TYPE
|
|
{
|
|
WINED3DBACKBUFFER_TYPE_MONO = 0,
|
|
WINED3DBACKBUFFER_TYPE_LEFT = 1,
|
|
WINED3DBACKBUFFER_TYPE_RIGHT = 2,
|
|
WINED3DBACKBUFFER_TYPE_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DBACKBUFFER_TYPE;
|
|
|
|
typedef enum _WINED3DSWAPEFFECT
|
|
{
|
|
WINED3DSWAPEFFECT_DISCARD = 1,
|
|
WINED3DSWAPEFFECT_FLIP = 2,
|
|
WINED3DSWAPEFFECT_COPY = 3,
|
|
WINED3DSWAPEFFECT_COPY_VSYNC = 4,
|
|
WINED3DSWAPEFFECT_FORCE_DWORD = 0xffffffff
|
|
} WINED3DSWAPEFFECT;
|
|
|
|
typedef enum _WINED3DSAMPLERSTATETYPE
|
|
{
|
|
WINED3DSAMP_ADDRESSU = 1,
|
|
WINED3DSAMP_ADDRESSV = 2,
|
|
WINED3DSAMP_ADDRESSW = 3,
|
|
WINED3DSAMP_BORDERCOLOR = 4,
|
|
WINED3DSAMP_MAGFILTER = 5,
|
|
WINED3DSAMP_MINFILTER = 6,
|
|
WINED3DSAMP_MIPFILTER = 7,
|
|
WINED3DSAMP_MIPMAPLODBIAS = 8,
|
|
WINED3DSAMP_MAXMIPLEVEL = 9,
|
|
WINED3DSAMP_MAXANISOTROPY = 10,
|
|
WINED3DSAMP_SRGBTEXTURE = 11,
|
|
WINED3DSAMP_ELEMENTINDEX = 12,
|
|
WINED3DSAMP_DMAPOFFSET = 13,
|
|
WINED3DSAMP_FORCE_DWORD = 0x7fffffff,
|
|
} WINED3DSAMPLERSTATETYPE;
|
|
const UINT WINED3D_HIGHEST_SAMPLER_STATE = WINED3DSAMP_DMAPOFFSET;
|
|
|
|
typedef enum _WINED3DMULTISAMPLE_TYPE
|
|
{
|
|
WINED3DMULTISAMPLE_NONE = 0,
|
|
WINED3DMULTISAMPLE_NONMASKABLE = 1,
|
|
WINED3DMULTISAMPLE_2_SAMPLES = 2,
|
|
WINED3DMULTISAMPLE_3_SAMPLES = 3,
|
|
WINED3DMULTISAMPLE_4_SAMPLES = 4,
|
|
WINED3DMULTISAMPLE_5_SAMPLES = 5,
|
|
WINED3DMULTISAMPLE_6_SAMPLES = 6,
|
|
WINED3DMULTISAMPLE_7_SAMPLES = 7,
|
|
WINED3DMULTISAMPLE_8_SAMPLES = 8,
|
|
WINED3DMULTISAMPLE_9_SAMPLES = 9,
|
|
WINED3DMULTISAMPLE_10_SAMPLES = 10,
|
|
WINED3DMULTISAMPLE_11_SAMPLES = 11,
|
|
WINED3DMULTISAMPLE_12_SAMPLES = 12,
|
|
WINED3DMULTISAMPLE_13_SAMPLES = 13,
|
|
WINED3DMULTISAMPLE_14_SAMPLES = 14,
|
|
WINED3DMULTISAMPLE_15_SAMPLES = 15,
|
|
WINED3DMULTISAMPLE_16_SAMPLES = 16,
|
|
WINED3DMULTISAMPLE_FORCE_DWORD = 0xffffffff
|
|
} WINED3DMULTISAMPLE_TYPE;
|
|
|
|
typedef enum _WINED3DTEXTURESTAGESTATETYPE
|
|
{
|
|
WINED3DTSS_COLOROP = 0,
|
|
WINED3DTSS_COLORARG1 = 1,
|
|
WINED3DTSS_COLORARG2 = 2,
|
|
WINED3DTSS_ALPHAOP = 3,
|
|
WINED3DTSS_ALPHAARG1 = 4,
|
|
WINED3DTSS_ALPHAARG2 = 5,
|
|
WINED3DTSS_BUMPENVMAT00 = 6,
|
|
WINED3DTSS_BUMPENVMAT01 = 7,
|
|
WINED3DTSS_BUMPENVMAT10 = 8,
|
|
WINED3DTSS_BUMPENVMAT11 = 9,
|
|
WINED3DTSS_TEXCOORDINDEX = 10,
|
|
WINED3DTSS_BUMPENVLSCALE = 11,
|
|
WINED3DTSS_BUMPENVLOFFSET = 12,
|
|
WINED3DTSS_TEXTURETRANSFORMFLAGS = 13,
|
|
WINED3DTSS_COLORARG0 = 14,
|
|
WINED3DTSS_ALPHAARG0 = 15,
|
|
WINED3DTSS_RESULTARG = 16,
|
|
WINED3DTSS_CONSTANT = 17,
|
|
WINED3DTSS_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DTEXTURESTAGESTATETYPE;
|
|
const UINT WINED3D_HIGHEST_TEXTURE_STATE = WINED3DTSS_CONSTANT;
|
|
|
|
typedef enum _WINED3DTEXTURETRANSFORMFLAGS
|
|
{
|
|
WINED3DTTFF_DISABLE = 0,
|
|
WINED3DTTFF_COUNT1 = 1,
|
|
WINED3DTTFF_COUNT2 = 2,
|
|
WINED3DTTFF_COUNT3 = 3,
|
|
WINED3DTTFF_COUNT4 = 4,
|
|
WINED3DTTFF_PROJECTED = 256,
|
|
WINED3DTTFF_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DTEXTURETRANSFORMFLAGS;
|
|
|
|
typedef enum _WINED3DTEXTUREOP
|
|
{
|
|
WINED3DTOP_DISABLE = 1,
|
|
WINED3DTOP_SELECTARG1 = 2,
|
|
WINED3DTOP_SELECTARG2 = 3,
|
|
WINED3DTOP_MODULATE = 4,
|
|
WINED3DTOP_MODULATE2X = 5,
|
|
WINED3DTOP_MODULATE4X = 6,
|
|
WINED3DTOP_ADD = 7,
|
|
WINED3DTOP_ADDSIGNED = 8,
|
|
WINED3DTOP_ADDSIGNED2X = 9,
|
|
WINED3DTOP_SUBTRACT = 10,
|
|
WINED3DTOP_ADDSMOOTH = 11,
|
|
WINED3DTOP_BLENDDIFFUSEALPHA = 12,
|
|
WINED3DTOP_BLENDTEXTUREALPHA = 13,
|
|
WINED3DTOP_BLENDFACTORALPHA = 14,
|
|
WINED3DTOP_BLENDTEXTUREALPHAPM = 15,
|
|
WINED3DTOP_BLENDCURRENTALPHA = 16,
|
|
WINED3DTOP_PREMODULATE = 17,
|
|
WINED3DTOP_MODULATEALPHA_ADDCOLOR = 18,
|
|
WINED3DTOP_MODULATECOLOR_ADDALPHA = 19,
|
|
WINED3DTOP_MODULATEINVALPHA_ADDCOLOR = 20,
|
|
WINED3DTOP_MODULATEINVCOLOR_ADDALPHA = 21,
|
|
WINED3DTOP_BUMPENVMAP = 22,
|
|
WINED3DTOP_BUMPENVMAPLUMINANCE = 23,
|
|
WINED3DTOP_DOTPRODUCT3 = 24,
|
|
WINED3DTOP_MULTIPLYADD = 25,
|
|
WINED3DTOP_LERP = 26,
|
|
WINED3DTOP_FORCE_DWORD = 0x7fffffff,
|
|
} WINED3DTEXTUREOP;
|
|
|
|
typedef enum _WINED3DTEXTUREADDRESS
|
|
{
|
|
WINED3DTADDRESS_WRAP = 1,
|
|
WINED3DTADDRESS_MIRROR = 2,
|
|
WINED3DTADDRESS_CLAMP = 3,
|
|
WINED3DTADDRESS_BORDER = 4,
|
|
WINED3DTADDRESS_MIRRORONCE = 5,
|
|
WINED3DTADDRESS_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DTEXTUREADDRESS;
|
|
|
|
typedef enum _WINED3DTRANSFORMSTATETYPE
|
|
{
|
|
WINED3DTS_VIEW = 2,
|
|
WINED3DTS_PROJECTION = 3,
|
|
WINED3DTS_TEXTURE0 = 16,
|
|
WINED3DTS_TEXTURE1 = 17,
|
|
WINED3DTS_TEXTURE2 = 18,
|
|
WINED3DTS_TEXTURE3 = 19,
|
|
WINED3DTS_TEXTURE4 = 20,
|
|
WINED3DTS_TEXTURE5 = 21,
|
|
WINED3DTS_TEXTURE6 = 22,
|
|
WINED3DTS_TEXTURE7 = 23,
|
|
WINED3DTS_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DTRANSFORMSTATETYPE;
|
|
cpp_quote("#define WINED3DTS_WORLD WINED3DTS_WORLDMATRIX(0)")
|
|
cpp_quote("#define WINED3DTS_WORLD1 WINED3DTS_WORLDMATRIX(1)")
|
|
cpp_quote("#define WINED3DTS_WORLD2 WINED3DTS_WORLDMATRIX(2)")
|
|
cpp_quote("#define WINED3DTS_WORLD3 WINED3DTS_WORLDMATRIX(3)")
|
|
cpp_quote("#define WINED3DTS_WORLDMATRIX(index) (WINED3DTRANSFORMSTATETYPE)(index + 256)")
|
|
|
|
typedef enum _WINED3DBASISTYPE
|
|
{
|
|
WINED3DBASIS_BEZIER = 0,
|
|
WINED3DBASIS_BSPLINE = 1,
|
|
WINED3DBASIS_INTERPOLATE = 2,
|
|
WINED3DBASIS_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DBASISTYPE;
|
|
|
|
typedef enum _WINED3DCUBEMAP_FACES
|
|
{
|
|
WINED3DCUBEMAP_FACE_POSITIVE_X = 0,
|
|
WINED3DCUBEMAP_FACE_NEGATIVE_X = 1,
|
|
WINED3DCUBEMAP_FACE_POSITIVE_Y = 2,
|
|
WINED3DCUBEMAP_FACE_NEGATIVE_Y = 3,
|
|
WINED3DCUBEMAP_FACE_POSITIVE_Z = 4,
|
|
WINED3DCUBEMAP_FACE_NEGATIVE_Z = 5,
|
|
WINED3DCUBEMAP_FACE_FORCE_DWORD = 0xffffffff
|
|
} WINED3DCUBEMAP_FACES;
|
|
|
|
typedef enum _WINED3DTEXTUREFILTERTYPE
|
|
{
|
|
WINED3DTEXF_NONE = 0,
|
|
WINED3DTEXF_POINT = 1,
|
|
WINED3DTEXF_LINEAR = 2,
|
|
WINED3DTEXF_ANISOTROPIC = 3,
|
|
WINED3DTEXF_FLATCUBIC = 4,
|
|
WINED3DTEXF_GAUSSIANCUBIC = 5,
|
|
WINED3DTEXF_PYRAMIDALQUAD = 6,
|
|
WINED3DTEXF_GAUSSIANQUAD = 7,
|
|
WINED3DTEXF_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DTEXTUREFILTERTYPE;
|
|
|
|
typedef enum _WINED3DRESOURCETYPE
|
|
{
|
|
WINED3DRTYPE_SURFACE = 1,
|
|
WINED3DRTYPE_VOLUME = 2,
|
|
WINED3DRTYPE_TEXTURE = 3,
|
|
WINED3DRTYPE_VOLUMETEXTURE = 4,
|
|
WINED3DRTYPE_CUBETEXTURE = 5,
|
|
WINED3DRTYPE_VERTEXBUFFER = 6,
|
|
WINED3DRTYPE_INDEXBUFFER = 7,
|
|
WINED3DRTYPE_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DRESOURCETYPE;
|
|
const UINT WINED3DRTYPECOUNT = WINED3DRTYPE_INDEXBUFFER + 1;
|
|
|
|
typedef enum _WINED3DPOOL
|
|
{
|
|
WINED3DPOOL_DEFAULT = 0,
|
|
WINED3DPOOL_MANAGED = 1,
|
|
WINED3DPOOL_SYSTEMMEM = 2,
|
|
WINED3DPOOL_SCRATCH = 3,
|
|
WINED3DPOOL_FORCE_DWORD = 0x7fffffff
|
|
} WINED3DPOOL;
|
|
|
|
typedef enum _WINED3DQUERYTYPE
|
|
{
|
|
WINED3DQUERYTYPE_VCACHE = 4,
|
|
WINED3DQUERYTYPE_RESOURCEMANAGER = 5,
|
|
WINED3DQUERYTYPE_VERTEXSTATS = 6,
|
|
WINED3DQUERYTYPE_EVENT = 8,
|
|
WINED3DQUERYTYPE_OCCLUSION = 9,
|
|
WINED3DQUERYTYPE_TIMESTAMP = 10,
|
|
WINED3DQUERYTYPE_TIMESTAMPDISJOINT = 11,
|
|
WINED3DQUERYTYPE_TIMESTAMPFREQ = 12,
|
|
WINED3DQUERYTYPE_PIPELINETIMINGS = 13,
|
|
WINED3DQUERYTYPE_INTERFACETIMINGS = 14,
|
|
WINED3DQUERYTYPE_VERTEXTIMINGS = 15,
|
|
WINED3DQUERYTYPE_PIXELTIMINGS = 16,
|
|
WINED3DQUERYTYPE_BANDWIDTHTIMINGS = 17,
|
|
WINED3DQUERYTYPE_CACHEUTILIZATION = 18
|
|
} WINED3DQUERYTYPE;
|
|
|
|
const UINT WINED3DISSUE_BEGIN = (1 << 1);
|
|
const UINT WINED3DISSUE_END = (1 << 0);
|
|
const UINT WINED3DGETDATA_FLUSH = (1 << 0);
|
|
|
|
typedef enum _WINED3DSTATEBLOCKTYPE
|
|
{
|
|
WINED3DSBT_INIT = 0,
|
|
WINED3DSBT_ALL = 1,
|
|
WINED3DSBT_PIXELSTATE = 2,
|
|
WINED3DSBT_VERTEXSTATE = 3,
|
|
WINED3DSBT_RECORDED = 4, /* WineD3D private */
|
|
WINED3DSBT_FORCE_DWORD = 0xffffffff
|
|
} WINED3DSTATEBLOCKTYPE;
|
|
|
|
typedef enum _WINED3DDECLMETHOD
|
|
{
|
|
WINED3DDECLMETHOD_DEFAULT = 0,
|
|
WINED3DDECLMETHOD_PARTIALU = 1,
|
|
WINED3DDECLMETHOD_PARTIALV = 2,
|
|
WINED3DDECLMETHOD_CROSSUV = 3,
|
|
WINED3DDECLMETHOD_UV = 4,
|
|
WINED3DDECLMETHOD_LOOKUP = 5,
|
|
WINED3DDECLMETHOD_LOOKUPPRESAMPLED = 6
|
|
} WINED3DDECLMETHOD;
|
|
|
|
typedef enum _WINED3DDECLTYPE
|
|
{
|
|
WINED3DDECLTYPE_FLOAT1 = 0,
|
|
WINED3DDECLTYPE_FLOAT2 = 1,
|
|
WINED3DDECLTYPE_FLOAT3 = 2,
|
|
WINED3DDECLTYPE_FLOAT4 = 3,
|
|
WINED3DDECLTYPE_D3DCOLOR = 4,
|
|
WINED3DDECLTYPE_UBYTE4 = 5,
|
|
WINED3DDECLTYPE_SHORT2 = 6,
|
|
WINED3DDECLTYPE_SHORT4 = 7,
|
|
/* VS 2.0 */
|
|
WINED3DDECLTYPE_UBYTE4N = 8,
|
|
WINED3DDECLTYPE_SHORT2N = 9,
|
|
WINED3DDECLTYPE_SHORT4N = 10,
|
|
WINED3DDECLTYPE_USHORT2N = 11,
|
|
WINED3DDECLTYPE_USHORT4N = 12,
|
|
WINED3DDECLTYPE_UDEC3 = 13,
|
|
WINED3DDECLTYPE_DEC3N = 14,
|
|
WINED3DDECLTYPE_FLOAT16_2 = 15,
|
|
WINED3DDECLTYPE_FLOAT16_4 = 16,
|
|
WINED3DDECLTYPE_UNUSED = 17,
|
|
} WINED3DDECLTYPE;
|
|
cpp_quote("#define WINED3DDECL_END() {0xFF, 0, WINED3DDECLTYPE_UNUSED, 0, 0, 0, -1}")
|
|
|
|
typedef enum _WINED3DDECLUSAGE
|
|
{
|
|
WINED3DDECLUSAGE_POSITION = 0,
|
|
WINED3DDECLUSAGE_BLENDWEIGHT = 1,
|
|
WINED3DDECLUSAGE_BLENDINDICES = 2,
|
|
WINED3DDECLUSAGE_NORMAL = 3,
|
|
WINED3DDECLUSAGE_PSIZE = 4,
|
|
WINED3DDECLUSAGE_TEXCOORD = 5,
|
|
WINED3DDECLUSAGE_TANGENT = 6,
|
|
WINED3DDECLUSAGE_BINORMAL = 7,
|
|
WINED3DDECLUSAGE_TESSFACTOR = 8,
|
|
WINED3DDECLUSAGE_POSITIONT = 9,
|
|
WINED3DDECLUSAGE_COLOR = 10,
|
|
WINED3DDECLUSAGE_FOG = 11,
|
|
WINED3DDECLUSAGE_DEPTH = 12,
|
|
WINED3DDECLUSAGE_SAMPLE = 13
|
|
} WINED3DDECLUSAGE;
|
|
|
|
typedef enum _WINED3DSURFTYPE
|
|
{
|
|
SURFACE_UNKNOWN = 0, /* Default / Unknown surface type */
|
|
SURFACE_OPENGL, /* OpenGL surface: Renders using libGL, needed for 3D */
|
|
SURFACE_GDI, /* User surface. No 3D, DirectDraw rendering with GDI */
|
|
} WINED3DSURFTYPE;
|
|
|
|
const UINT WINED3DCOLORWRITEENABLE_RED = (1<<0);
|
|
const UINT WINED3DCOLORWRITEENABLE_GREEN = (1<<1);
|
|
const UINT WINED3DCOLORWRITEENABLE_BLUE = (1<<2);
|
|
const UINT WINED3DCOLORWRITEENABLE_ALPHA = (1<<3);
|
|
|
|
const UINT WINED3DADAPTER_DEFAULT = 0;
|
|
const UINT WINED3DENUM_NO_WHQL_LEVEL = 2;
|
|
const UINT WINED3DPRESENT_BACK_BUFFER_MAX = 3;
|
|
|
|
const UINT WINED3DTSS_TCI_PASSTHRU = 0x00000;
|
|
const UINT WINED3DTSS_TCI_CAMERASPACENORMAL = 0x10000;
|
|
const UINT WINED3DTSS_TCI_CAMERASPACEPOSITION = 0x20000;
|
|
const UINT WINED3DTSS_TCI_CAMERASPACEREFLECTIONVECTOR = 0x30000;
|
|
const UINT WINED3DTSS_TCI_SPHEREMAP = 0x40000;
|
|
|
|
const UINT WINED3DTA_SELECTMASK = 0x0000000f;
|
|
const UINT WINED3DTA_DIFFUSE = 0x00000000;
|
|
const UINT WINED3DTA_CURRENT = 0x00000001;
|
|
const UINT WINED3DTA_TEXTURE = 0x00000002;
|
|
const UINT WINED3DTA_TFACTOR = 0x00000003;
|
|
const UINT WINED3DTA_SPECULAR = 0x00000004;
|
|
const UINT WINED3DTA_TEMP = 0x00000005;
|
|
const UINT WINED3DTA_CONSTANT = 0x00000006;
|
|
const UINT WINED3DTA_COMPLEMENT = 0x00000010;
|
|
const UINT WINED3DTA_ALPHAREPLICATE = 0x00000020;
|
|
|
|
const UINT WINED3DPRESENTFLAG_LOCKABLE_BACKBUFFER = 0x00000001;
|
|
const UINT WINED3DPRESENTFLAG_DISCARD_DEPTHSTENCIL = 0x00000002;
|
|
const UINT WINED3DPRESENTFLAG_DEVICECLIP = 0x00000004;
|
|
const UINT WINED3DPRESENTFLAG_VIDEO = 0x00000010;
|
|
const UINT WINED3DPRESENTFLAG_NOAUTOROTATE = 0x00000020;
|
|
const UINT WINED3DPRESENTFLAG_UNPRUNEDMODE = 0x00000040;
|
|
|
|
const UINT WINED3DDP_MAXTEXCOORD = 8;
|
|
|
|
const UINT WINED3DUSAGE_RENDERTARGET = 0x00000001;
|
|
const UINT WINED3DUSAGE_DEPTHSTENCIL = 0x00000002;
|
|
const UINT WINED3DUSAGE_WRITEONLY = 0x00000008;
|
|
const UINT WINED3DUSAGE_SOFTWAREPROCESSING = 0x00000010;
|
|
const UINT WINED3DUSAGE_DONOTCLIP = 0x00000020;
|
|
const UINT WINED3DUSAGE_POINTS = 0x00000040;
|
|
const UINT WINED3DUSAGE_RTPATCHES = 0x00000080;
|
|
const UINT WINED3DUSAGE_NPATCHES = 0x00000100;
|
|
const UINT WINED3DUSAGE_DYNAMIC = 0x00000200;
|
|
const UINT WINED3DUSAGE_AUTOGENMIPMAP = 0x00000400;
|
|
const UINT WINED3DUSAGE_DMAP = 0x00004000;
|
|
const UINT WINED3DUSAGE_MASK = 0x00004fff;
|
|
const UINT WINED3DUSAGE_OVERLAY = 0x80000000;
|
|
|
|
const UINT WINED3DUSAGE_QUERY_LEGACYBUMPMAP = 0x00008000;
|
|
const UINT WINED3DUSAGE_QUERY_FILTER = 0x00020000;
|
|
const UINT WINED3DUSAGE_QUERY_POSTPIXELSHADER_BLENDING = 0x00080000;
|
|
const UINT WINED3DUSAGE_QUERY_SRGBREAD = 0x00010000;
|
|
const UINT WINED3DUSAGE_QUERY_SRGBWRITE = 0x00040000;
|
|
const UINT WINED3DUSAGE_QUERY_VERTEXTEXTURE = 0x00100000;
|
|
const UINT WINED3DUSAGE_QUERY_WRAPANDMIP = 0x00200000;
|
|
const UINT WINED3DUSAGE_QUERY_MASK = 0x003f8000;
|
|
|
|
const UINT WINED3DLOCK_READONLY = 0x0010;
|
|
const UINT WINED3DLOCK_NOSYSLOCK = 0x0800;
|
|
const UINT WINED3DLOCK_NOOVERWRITE = 0x1000;
|
|
const UINT WINED3DLOCK_DISCARD = 0x2000;
|
|
const UINT WINED3DLOCK_DONOTWAIT = 0x4000;
|
|
const UINT WINED3DLOCK_NO_DIRTY_UPDATE = 0x8000;
|
|
|
|
const UINT WINED3DPRESENT_RATE_DEFAULT = 0x000000000;
|
|
|
|
const UINT WINED3DPRESENT_INTERVAL_DEFAULT = 0x00000000;
|
|
const UINT WINED3DPRESENT_INTERVAL_ONE = 0x00000001;
|
|
const UINT WINED3DPRESENT_INTERVAL_TWO = 0x00000002;
|
|
const UINT WINED3DPRESENT_INTERVAL_THREE = 0x00000004;
|
|
const UINT WINED3DPRESENT_INTERVAL_FOUR = 0x00000008;
|
|
const UINT WINED3DPRESENT_INTERVAL_IMMEDIATE = 0x80000000;
|
|
|
|
const UINT WINED3DMAXUSERCLIPPLANES = 32;
|
|
const UINT WINED3DCLIPPLANE0 = (1 << 0);
|
|
const UINT WINED3DCLIPPLANE1 = (1 << 1);
|
|
const UINT WINED3DCLIPPLANE2 = (1 << 2);
|
|
const UINT WINED3DCLIPPLANE3 = (1 << 3);
|
|
const UINT WINED3DCLIPPLANE4 = (1 << 4);
|
|
const UINT WINED3DCLIPPLANE5 = (1 << 5);
|
|
|
|
/* FVF (Flexible Vertex Format) codes */
|
|
const UINT WINED3DFVF_RESERVED0 = 0x0001;
|
|
const UINT WINED3DFVF_POSITION_MASK = 0x400e;
|
|
const UINT WINED3DFVF_XYZ = 0x0002;
|
|
const UINT WINED3DFVF_XYZRHW = 0x0004;
|
|
const UINT WINED3DFVF_XYZB1 = 0x0006;
|
|
const UINT WINED3DFVF_XYZB2 = 0x0008;
|
|
const UINT WINED3DFVF_XYZB3 = 0x000a;
|
|
const UINT WINED3DFVF_XYZB4 = 0x000c;
|
|
const UINT WINED3DFVF_XYZB5 = 0x000e;
|
|
const UINT WINED3DFVF_XYZW = 0x4002;
|
|
const UINT WINED3DFVF_NORMAL = 0x0010;
|
|
const UINT WINED3DFVF_PSIZE = 0x0020;
|
|
const UINT WINED3DFVF_DIFFUSE = 0x0040;
|
|
const UINT WINED3DFVF_SPECULAR = 0x0080;
|
|
const UINT WINED3DFVF_TEXCOUNT_MASK = 0x0f00;
|
|
const UINT WINED3DFVF_TEXCOUNT_SHIFT = 8;
|
|
const UINT WINED3DFVF_TEX0 = 0x0000;
|
|
const UINT WINED3DFVF_TEX1 = 0x0100;
|
|
const UINT WINED3DFVF_TEX2 = 0x0200;
|
|
const UINT WINED3DFVF_TEX3 = 0x0300;
|
|
const UINT WINED3DFVF_TEX4 = 0x0400;
|
|
const UINT WINED3DFVF_TEX5 = 0x0500;
|
|
const UINT WINED3DFVF_TEX6 = 0x0600;
|
|
const UINT WINED3DFVF_TEX7 = 0x0700;
|
|
const UINT WINED3DFVF_TEX8 = 0x0800;
|
|
const UINT WINED3DFVF_LASTBETA_UBYTE4 = 0x1000;
|
|
const UINT WINED3DFVF_LASTBETA_D3DCOLOR = 0x8000;
|
|
const UINT WINED3DFVF_RESERVED2 = 0x6000;
|
|
|
|
const UINT WINED3DFVF_TEXTUREFORMAT1 = 3;
|
|
const UINT WINED3DFVF_TEXTUREFORMAT2 = 0;
|
|
const UINT WINED3DFVF_TEXTUREFORMAT3 = 1;
|
|
const UINT WINED3DFVF_TEXTUREFORMAT4 = 2;
|
|
cpp_quote("#define WINED3DFVF_TEXCOORDSIZE1(CoordIndex) (WINED3DFVF_TEXTUREFORMAT1 << (CoordIndex*2 + 16))")
|
|
cpp_quote("#define WINED3DFVF_TEXCOORDSIZE2(CoordIndex) (WINED3DFVF_TEXTUREFORMAT2)")
|
|
cpp_quote("#define WINED3DFVF_TEXCOORDSIZE3(CoordIndex) (WINED3DFVF_TEXTUREFORMAT3 << (CoordIndex*2 + 16))")
|
|
cpp_quote("#define WINED3DFVF_TEXCOORDSIZE4(CoordIndex) (WINED3DFVF_TEXTUREFORMAT4 << (CoordIndex*2 + 16))")
|
|
|
|
/* Clear flags */
|
|
const UINT WINED3DCLEAR_TARGET = 0x00000001;
|
|
const UINT WINED3DCLEAR_ZBUFFER = 0x00000002;
|
|
const UINT WINED3DCLEAR_STENCIL = 0x00000004;
|
|
|
|
/* Stream source flags */
|
|
const UINT WINED3DSTREAMSOURCE_INDEXEDDATA = (1 << 30);
|
|
const UINT WINED3DSTREAMSOURCE_INSTANCEDATA = (2 << 30);
|
|
|
|
/* SetPrivateData flags */
|
|
const UINT WINED3DSPD_IUNKNOWN = 0x00000001;
|
|
|
|
/* IWineD3D::CreateDevice behaviour flags */
|
|
const UINT WINED3DCREATE_FPU_PRESERVE = 0x00000002;
|
|
const UINT WINED3DCREATE_PUREDEVICE = 0x00000010;
|
|
const UINT WINED3DCREATE_SOFTWARE_VERTEXPROCESSING = 0x00000020;
|
|
const UINT WINED3DCREATE_HARDWARE_VERTEXPROCESSING = 0x00000040;
|
|
const UINT WINED3DCREATE_MIXED_VERTEXPROCESSING = 0x00000080;
|
|
const UINT WINED3DCREATE_DISABLE_DRIVER_MANAGEMENT = 0x00000100;
|
|
const UINT WINED3DCREATE_ADAPTERGROUP_DEVICE = 0x00000200;
|
|
|
|
/* VTF defines */
|
|
const UINT WINED3DDMAPSAMPLER = 0x100;
|
|
const UINT WINED3DVERTEXTEXTURESAMPLER0 = (WINED3DDMAPSAMPLER + 1);
|
|
const UINT WINED3DVERTEXTEXTURESAMPLER1 = (WINED3DDMAPSAMPLER + 2);
|
|
const UINT WINED3DVERTEXTEXTURESAMPLER2 = (WINED3DDMAPSAMPLER + 3);
|
|
const UINT WINED3DVERTEXTEXTURESAMPLER3 = (WINED3DDMAPSAMPLER + 4);
|
|
|
|
const UINT WINED3DCAPS3_ALPHA_FULLSCREEN_FLIP_OR_DISCARD = 0x00000020;
|
|
const UINT WINED3DCAPS3_LINEAR_TO_SRGB_PRESENTATION = 0x00000080;
|
|
const UINT WINED3DCAPS3_COPY_TO_VIDMEM = 0x00000100;
|
|
const UINT WINED3DCAPS3_COPY_TO_SYSTEMMEM = 0x00000200;
|
|
const UINT WINED3DCAPS3_RESERVED = 0x8000001f;
|
|
|
|
const UINT WINED3DDEVCAPS2_STREAMOFFSET = 0x00000001;
|
|
const UINT WINED3DDEVCAPS2_DMAPNPATCH = 0x00000002;
|
|
const UINT WINED3DDEVCAPS2_ADAPTIVETESSRTPATCH = 0x00000004;
|
|
const UINT WINED3DDEVCAPS2_ADAPTIVETESSNPATCH = 0x00000008;
|
|
const UINT WINED3DDEVCAPS2_CAN_STRETCHRECT_FROM_TEXTURES = 0x00000010;
|
|
const UINT WINED3DDEVCAPS2_PRESAMPLEDDMAPNPATCH = 0x00000020;
|
|
const UINT WINED3DDEVCAPS2_VERTEXELEMENTSCANSHARESTREAMOFFSET = 0x00000040;
|
|
|
|
const UINT WINED3DDTCAPS_UBYTE4 = 0x00000001;
|
|
const UINT WINED3DDTCAPS_UBYTE4N = 0x00000002;
|
|
const UINT WINED3DDTCAPS_SHORT2N = 0x00000004;
|
|
const UINT WINED3DDTCAPS_SHORT4N = 0x00000008;
|
|
const UINT WINED3DDTCAPS_USHORT2N = 0x00000010;
|
|
const UINT WINED3DDTCAPS_USHORT4N = 0x00000020;
|
|
const UINT WINED3DDTCAPS_UDEC3 = 0x00000040;
|
|
const UINT WINED3DDTCAPS_DEC3N = 0x00000080;
|
|
const UINT WINED3DDTCAPS_FLOAT16_2 = 0x00000100;
|
|
const UINT WINED3DDTCAPS_FLOAT16_4 = 0x00000200;
|
|
|
|
const UINT WINED3DFVFCAPS_TEXCOORDCOUNTMASK = 0x0000ffff;
|
|
const UINT WINED3DFVFCAPS_DONOTSTRIPELEMENTS = 0x00080000;
|
|
const UINT WINED3DFVFCAPS_PSIZE = 0x00100000;
|
|
|
|
const UINT WINED3DLINECAPS_TEXTURE = 0x00000001;
|
|
const UINT WINED3DLINECAPS_ZTEST = 0x00000002;
|
|
const UINT WINED3DLINECAPS_BLEND = 0x00000004;
|
|
const UINT WINED3DLINECAPS_ALPHACMP = 0x00000008;
|
|
const UINT WINED3DLINECAPS_FOG = 0x00000010;
|
|
const UINT WINED3DLINECAPS_ANTIALIAS = 0x00000020;
|
|
|
|
const UINT WINED3DMAX30SHADERINSTRUCTIONS = 32768;
|
|
const UINT WINED3DMIN30SHADERINSTRUCTIONS = 512;
|
|
|
|
const UINT WINED3DPBLENDCAPS_ZERO = 0x00000001;
|
|
const UINT WINED3DPBLENDCAPS_ONE = 0x00000002;
|
|
const UINT WINED3DPBLENDCAPS_SRCCOLOR = 0x00000004;
|
|
const UINT WINED3DPBLENDCAPS_INVSRCCOLOR = 0x00000008;
|
|
const UINT WINED3DPBLENDCAPS_SRCALPHA = 0x00000010;
|
|
const UINT WINED3DPBLENDCAPS_INVSRCALPHA = 0x00000020;
|
|
const UINT WINED3DPBLENDCAPS_DESTALPHA = 0x00000040;
|
|
const UINT WINED3DPBLENDCAPS_INVDESTALPHA = 0x00000080;
|
|
const UINT WINED3DPBLENDCAPS_DESTCOLOR = 0x00000100;
|
|
const UINT WINED3DPBLENDCAPS_INVDESTCOLOR = 0x00000200;
|
|
const UINT WINED3DPBLENDCAPS_SRCALPHASAT = 0x00000400;
|
|
const UINT WINED3DPBLENDCAPS_BOTHSRCALPHA = 0x00000800;
|
|
const UINT WINED3DPBLENDCAPS_BOTHINVSRCALPHA = 0x00001000;
|
|
const UINT WINED3DPBLENDCAPS_BLENDFACTOR = 0x00002000;
|
|
|
|
const UINT WINED3DPCMPCAPS_NEVER = 0x00000001;
|
|
const UINT WINED3DPCMPCAPS_LESS = 0x00000002;
|
|
const UINT WINED3DPCMPCAPS_EQUAL = 0x00000004;
|
|
const UINT WINED3DPCMPCAPS_LESSEQUAL = 0x00000008;
|
|
const UINT WINED3DPCMPCAPS_GREATER = 0x00000010;
|
|
const UINT WINED3DPCMPCAPS_NOTEQUAL = 0x00000020;
|
|
const UINT WINED3DPCMPCAPS_GREATEREQUAL = 0x00000040;
|
|
const UINT WINED3DPCMPCAPS_ALWAYS = 0x00000080;
|
|
|
|
const UINT WINED3DPMISCCAPS_MASKZ = 0x00000002;
|
|
const UINT WINED3DPMISCCAPS_LINEPATTERNREP = 0x00000004;
|
|
const UINT WINED3DPMISCCAPS_CULLNONE = 0x00000010;
|
|
const UINT WINED3DPMISCCAPS_CULLCW = 0x00000020;
|
|
const UINT WINED3DPMISCCAPS_CULLCCW = 0x00000040;
|
|
const UINT WINED3DPMISCCAPS_COLORWRITEENABLE = 0x00000080;
|
|
const UINT WINED3DPMISCCAPS_CLIPPLANESCALEDPOINTS = 0x00000100;
|
|
const UINT WINED3DPMISCCAPS_CLIPTLVERTS = 0x00000200;
|
|
const UINT WINED3DPMISCCAPS_TSSARGTEMP = 0x00000400;
|
|
const UINT WINED3DPMISCCAPS_BLENDOP = 0x00000800;
|
|
const UINT WINED3DPMISCCAPS_NULLREFERENCE = 0x00001000;
|
|
const UINT WINED3DPMISCCAPS_INDEPENDENTWRITEMASKS = 0x00004000;
|
|
const UINT WINED3DPMISCCAPS_PERSTAGECONSTANT = 0x00008000;
|
|
const UINT WINED3DPMISCCAPS_FOGANDSPECULARALPHA = 0x00010000;
|
|
const UINT WINED3DPMISCCAPS_SEPARATEALPHABLEND = 0x00020000;
|
|
const UINT WINED3DPMISCCAPS_MRTINDEPENDENTBITDEPTHS = 0x00040000;
|
|
const UINT WINED3DPMISCCAPS_MRTPOSTPIXELSHADERBLENDING = 0x00080000;
|
|
const UINT WINED3DPMISCCAPS_FOGVERTEXCLAMPED = 0x00100000;
|
|
|
|
const UINT WINED3DPS20_MAX_DYNAMICFLOWCONTROLDEPTH = 24;
|
|
const UINT WINED3DPS20_MIN_DYNAMICFLOWCONTROLDEPTH = 0;
|
|
const UINT WINED3DPS20_MAX_NUMTEMPS = 32;
|
|
const UINT WINED3DPS20_MIN_NUMTEMPS = 12;
|
|
const UINT WINED3DPS20_MAX_STATICFLOWCONTROLDEPTH = 4;
|
|
const UINT WINED3DPS20_MIN_STATICFLOWCONTROLDEPTH = 0;
|
|
const UINT WINED3DPS20_MAX_NUMINSTRUCTIONSLOTS = 512;
|
|
const UINT WINED3DPS20_MIN_NUMINSTRUCTIONSLOTS = 96;
|
|
|
|
const UINT WINED3DPS20CAPS_ARBITRARYSWIZZLE = 0x00000001;
|
|
const UINT WINED3DPS20CAPS_GRADIENTINSTRUCTIONS = 0x00000002;
|
|
const UINT WINED3DPS20CAPS_PREDICATION = 0x00000004;
|
|
const UINT WINED3DPS20CAPS_NODEPENDENTREADLIMIT = 0x00000008;
|
|
const UINT WINED3DPS20CAPS_NOTEXINSTRUCTIONLIMIT = 0x00000010;
|
|
|
|
const UINT WINED3DPTADDRESSCAPS_WRAP = 0x00000001;
|
|
const UINT WINED3DPTADDRESSCAPS_MIRROR = 0x00000002;
|
|
const UINT WINED3DPTADDRESSCAPS_CLAMP = 0x00000004;
|
|
const UINT WINED3DPTADDRESSCAPS_BORDER = 0x00000008;
|
|
const UINT WINED3DPTADDRESSCAPS_INDEPENDENTUV = 0x00000010;
|
|
const UINT WINED3DPTADDRESSCAPS_MIRRORONCE = 0x00000020;
|
|
|
|
const UINT WINED3DSTENCILCAPS_KEEP = 0x00000001;
|
|
const UINT WINED3DSTENCILCAPS_ZERO = 0x00000002;
|
|
const UINT WINED3DSTENCILCAPS_REPLACE = 0x00000004;
|
|
const UINT WINED3DSTENCILCAPS_INCRSAT = 0x00000008;
|
|
const UINT WINED3DSTENCILCAPS_DECRSAT = 0x00000010;
|
|
const UINT WINED3DSTENCILCAPS_INVERT = 0x00000020;
|
|
const UINT WINED3DSTENCILCAPS_INCR = 0x00000040;
|
|
const UINT WINED3DSTENCILCAPS_DECR = 0x00000080;
|
|
const UINT WINED3DSTENCILCAPS_TWOSIDED = 0x00000100;
|
|
|
|
const UINT WINED3DTEXOPCAPS_DISABLE = 0x00000001;
|
|
const UINT WINED3DTEXOPCAPS_SELECTARG1 = 0x00000002;
|
|
const UINT WINED3DTEXOPCAPS_SELECTARG2 = 0x00000004;
|
|
const UINT WINED3DTEXOPCAPS_MODULATE = 0x00000008;
|
|
const UINT WINED3DTEXOPCAPS_MODULATE2X = 0x00000010;
|
|
const UINT WINED3DTEXOPCAPS_MODULATE4X = 0x00000020;
|
|
const UINT WINED3DTEXOPCAPS_ADD = 0x00000040;
|
|
const UINT WINED3DTEXOPCAPS_ADDSIGNED = 0x00000080;
|
|
const UINT WINED3DTEXOPCAPS_ADDSIGNED2X = 0x00000100;
|
|
const UINT WINED3DTEXOPCAPS_SUBTRACT = 0x00000200;
|
|
const UINT WINED3DTEXOPCAPS_ADDSMOOTH = 0x00000400;
|
|
const UINT WINED3DTEXOPCAPS_BLENDDIFFUSEALPHA = 0x00000800;
|
|
const UINT WINED3DTEXOPCAPS_BLENDTEXTUREALPHA = 0x00001000;
|
|
const UINT WINED3DTEXOPCAPS_BLENDFACTORALPHA = 0x00002000;
|
|
const UINT WINED3DTEXOPCAPS_BLENDTEXTUREALPHAPM = 0x00004000;
|
|
const UINT WINED3DTEXOPCAPS_BLENDCURRENTALPHA = 0x00008000;
|
|
const UINT WINED3DTEXOPCAPS_PREMODULATE = 0x00010000;
|
|
const UINT WINED3DTEXOPCAPS_MODULATEALPHA_ADDCOLOR = 0x00020000;
|
|
const UINT WINED3DTEXOPCAPS_MODULATECOLOR_ADDALPHA = 0x00040000;
|
|
const UINT WINED3DTEXOPCAPS_MODULATEINVALPHA_ADDCOLOR = 0x00080000;
|
|
const UINT WINED3DTEXOPCAPS_MODULATEINVCOLOR_ADDALPHA = 0x00100000;
|
|
const UINT WINED3DTEXOPCAPS_BUMPENVMAP = 0x00200000;
|
|
const UINT WINED3DTEXOPCAPS_BUMPENVMAPLUMINANCE = 0x00400000;
|
|
const UINT WINED3DTEXOPCAPS_DOTPRODUCT3 = 0x00800000;
|
|
const UINT WINED3DTEXOPCAPS_MULTIPLYADD = 0x01000000;
|
|
const UINT WINED3DTEXOPCAPS_LERP = 0x02000000;
|
|
|
|
const UINT WINED3DVS20_MAX_DYNAMICFLOWCONTROLDEPTH = 24;
|
|
const UINT WINED3DVS20_MIN_DYNAMICFLOWCONTROLDEPTH = 0;
|
|
const UINT WINED3DVS20_MAX_NUMTEMPS = 32;
|
|
const UINT WINED3DVS20_MIN_NUMTEMPS = 12;
|
|
const UINT WINED3DVS20_MAX_STATICFLOWCONTROLDEPTH = 4;
|
|
const UINT WINED3DVS20_MIN_STATICFLOWCONTROLDEPTH = 1;
|
|
|
|
const UINT WINED3DVS20CAPS_PREDICATION = 0x00000001;
|
|
|
|
const UINT WINED3DCAPS2_NO2DDURING3DSCENE = 0x00000002;
|
|
const UINT WINED3DCAPS2_FULLSCREENGAMMA = 0x00020000;
|
|
const UINT WINED3DCAPS2_CANRENDERWINDOWED = 0x00080000;
|
|
const UINT WINED3DCAPS2_CANCALIBRATEGAMMA = 0x00100000;
|
|
const UINT WINED3DCAPS2_RESERVED = 0x02000000;
|
|
const UINT WINED3DCAPS2_CANMANAGERESOURCE = 0x10000000;
|
|
const UINT WINED3DCAPS2_DYNAMICTEXTURES = 0x20000000;
|
|
const UINT WINED3DCAPS2_CANAUTOGENMIPMAP = 0x40000000;
|
|
|
|
const UINT WINED3DPRASTERCAPS_DITHER = 0x00000001;
|
|
const UINT WINED3DPRASTERCAPS_ROP2 = 0x00000002;
|
|
const UINT WINED3DPRASTERCAPS_XOR = 0x00000004;
|
|
const UINT WINED3DPRASTERCAPS_PAT = 0x00000008;
|
|
const UINT WINED3DPRASTERCAPS_ZTEST = 0x00000010;
|
|
const UINT WINED3DPRASTERCAPS_SUBPIXEL = 0x00000020;
|
|
const UINT WINED3DPRASTERCAPS_SUBPIXELX = 0x00000040;
|
|
const UINT WINED3DPRASTERCAPS_FOGVERTEX = 0x00000080;
|
|
const UINT WINED3DPRASTERCAPS_FOGTABLE = 0x00000100;
|
|
const UINT WINED3DPRASTERCAPS_STIPPLE = 0x00000200;
|
|
const UINT WINED3DPRASTERCAPS_ANTIALIASSORTDEPENDENT = 0x00000400;
|
|
const UINT WINED3DPRASTERCAPS_ANTIALIASSORTINDEPENDENT = 0x00000800;
|
|
const UINT WINED3DPRASTERCAPS_ANTIALIASEDGES = 0x00001000;
|
|
const UINT WINED3DPRASTERCAPS_MIPMAPLODBIAS = 0x00002000;
|
|
const UINT WINED3DPRASTERCAPS_ZBIAS = 0x00004000;
|
|
const UINT WINED3DPRASTERCAPS_ZBUFFERLESSHSR = 0x00008000;
|
|
const UINT WINED3DPRASTERCAPS_FOGRANGE = 0x00010000;
|
|
const UINT WINED3DPRASTERCAPS_ANISOTROPY = 0x00020000;
|
|
const UINT WINED3DPRASTERCAPS_WBUFFER = 0x00040000;
|
|
const UINT WINED3DPRASTERCAPS_TRANSLUCENTSORTINDEPENDENT = 0x00080000;
|
|
const UINT WINED3DPRASTERCAPS_WFOG = 0x00100000;
|
|
const UINT WINED3DPRASTERCAPS_ZFOG = 0x00200000;
|
|
const UINT WINED3DPRASTERCAPS_COLORPERSPECTIVE = 0x00400000;
|
|
const UINT WINED3DPRASTERCAPS_SCISSORTEST = 0x01000000;
|
|
const UINT WINED3DPRASTERCAPS_SLOPESCALEDEPTHBIAS = 0x02000000;
|
|
const UINT WINED3DPRASTERCAPS_DEPTHBIAS = 0x04000000;
|
|
const UINT WINED3DPRASTERCAPS_MULTISAMPLE_TOGGLE = 0x08000000;
|
|
|
|
const UINT WINED3DPSHADECAPS_COLORFLATMONO = 0x00000001;
|
|
const UINT WINED3DPSHADECAPS_COLORFLATRGB = 0x00000002;
|
|
const UINT WINED3DPSHADECAPS_COLORGOURAUDMONO = 0x00000004;
|
|
const UINT WINED3DPSHADECAPS_COLORGOURAUDRGB = 0x00000008;
|
|
const UINT WINED3DPSHADECAPS_COLORPHONGMONO = 0x00000010;
|
|
const UINT WINED3DPSHADECAPS_COLORPHONGRGB = 0x00000020;
|
|
const UINT WINED3DPSHADECAPS_SPECULARFLATMONO = 0x00000040;
|
|
const UINT WINED3DPSHADECAPS_SPECULARFLATRGB = 0x00000080;
|
|
const UINT WINED3DPSHADECAPS_SPECULARGOURAUDMONO = 0x00000100;
|
|
const UINT WINED3DPSHADECAPS_SPECULARGOURAUDRGB = 0x00000200;
|
|
const UINT WINED3DPSHADECAPS_SPECULARPHONGMONO = 0x00000400;
|
|
const UINT WINED3DPSHADECAPS_SPECULARPHONGRGB = 0x00000800;
|
|
const UINT WINED3DPSHADECAPS_ALPHAFLATBLEND = 0x00001000;
|
|
const UINT WINED3DPSHADECAPS_ALPHAFLATSTIPPLED = 0x00002000;
|
|
const UINT WINED3DPSHADECAPS_ALPHAGOURAUDBLEND = 0x00004000;
|
|
const UINT WINED3DPSHADECAPS_ALPHAGOURAUDSTIPPLED = 0x00008000;
|
|
const UINT WINED3DPSHADECAPS_ALPHAPHONGBLEND = 0x00010000;
|
|
const UINT WINED3DPSHADECAPS_ALPHAPHONGSTIPPLED = 0x00020000;
|
|
const UINT WINED3DPSHADECAPS_FOGFLAT = 0x00040000;
|
|
const UINT WINED3DPSHADECAPS_FOGGOURAUD = 0x00080000;
|
|
const UINT WINED3DPSHADECAPS_FOGPHONG = 0x00100000;
|
|
|
|
const UINT WINED3DPTEXTURECAPS_PERSPECTIVE = 0x00000001;
|
|
const UINT WINED3DPTEXTURECAPS_POW2 = 0x00000002;
|
|
const UINT WINED3DPTEXTURECAPS_ALPHA = 0x00000004;
|
|
const UINT WINED3DPTEXTURECAPS_TRANSPARENCY = 0x00000008;
|
|
const UINT WINED3DPTEXTURECAPS_BORDER = 0x00000010;
|
|
const UINT WINED3DPTEXTURECAPS_SQUAREONLY = 0x00000020;
|
|
const UINT WINED3DPTEXTURECAPS_TEXREPEATNOTSCALEDBYSIZE = 0x00000040;
|
|
const UINT WINED3DPTEXTURECAPS_ALPHAPALETTE = 0x00000080;
|
|
const UINT WINED3DPTEXTURECAPS_NONPOW2CONDITIONAL = 0x00000100;
|
|
const UINT WINED3DPTEXTURECAPS_PROJECTED = 0x00000400;
|
|
const UINT WINED3DPTEXTURECAPS_CUBEMAP = 0x00000800;
|
|
const UINT WINED3DPTEXTURECAPS_COLORKEYBLEND = 0x00001000;
|
|
const UINT WINED3DPTEXTURECAPS_VOLUMEMAP = 0x00002000;
|
|
const UINT WINED3DPTEXTURECAPS_MIPMAP = 0x00004000;
|
|
const UINT WINED3DPTEXTURECAPS_MIPVOLUMEMAP = 0x00008000;
|
|
const UINT WINED3DPTEXTURECAPS_MIPCUBEMAP = 0x00010000;
|
|
const UINT WINED3DPTEXTURECAPS_CUBEMAP_POW2 = 0x00020000;
|
|
const UINT WINED3DPTEXTURECAPS_VOLUMEMAP_POW2 = 0x00040000;
|
|
const UINT WINED3DPTEXTURECAPS_NOPROJECTEDBUMPENV = 0x00200000;
|
|
|
|
const UINT WINED3DPTFILTERCAPS_NEAREST = 0x00000001;
|
|
const UINT WINED3DPTFILTERCAPS_LINEAR = 0x00000002;
|
|
const UINT WINED3DPTFILTERCAPS_MIPNEAREST = 0x00000004;
|
|
const UINT WINED3DPTFILTERCAPS_MIPLINEAR = 0x00000008;
|
|
const UINT WINED3DPTFILTERCAPS_LINEARMIPNEAREST = 0x00000010;
|
|
const UINT WINED3DPTFILTERCAPS_LINEARMIPLINEAR = 0x00000020;
|
|
const UINT WINED3DPTFILTERCAPS_MINFPOINT = 0x00000100;
|
|
const UINT WINED3DPTFILTERCAPS_MINFLINEAR = 0x00000200;
|
|
const UINT WINED3DPTFILTERCAPS_MINFANISOTROPIC = 0x00000400;
|
|
const UINT WINED3DPTFILTERCAPS_MIPFPOINT = 0x00010000;
|
|
const UINT WINED3DPTFILTERCAPS_MIPFLINEAR = 0x00020000;
|
|
const UINT WINED3DPTFILTERCAPS_MAGFPOINT = 0x01000000;
|
|
const UINT WINED3DPTFILTERCAPS_MAGFLINEAR = 0x02000000;
|
|
const UINT WINED3DPTFILTERCAPS_MAGFANISOTROPIC = 0x04000000;
|
|
const UINT WINED3DPTFILTERCAPS_MAGFPYRAMIDALQUAD = 0x08000000;
|
|
const UINT WINED3DPTFILTERCAPS_MAGFGAUSSIANQUAD = 0x10000000;
|
|
|
|
const UINT WINED3DVTXPCAPS_TEXGEN = 0x00000001;
|
|
const UINT WINED3DVTXPCAPS_MATERIALSOURCE7 = 0x00000002;
|
|
const UINT WINED3DVTXPCAPS_VERTEXFOG = 0x00000004;
|
|
const UINT WINED3DVTXPCAPS_DIRECTIONALLIGHTS = 0x00000008;
|
|
const UINT WINED3DVTXPCAPS_POSITIONALLIGHTS = 0x00000010;
|
|
const UINT WINED3DVTXPCAPS_LOCALVIEWER = 0x00000020;
|
|
const UINT WINED3DVTXPCAPS_TWEENING = 0x00000040;
|
|
const UINT WINED3DVTXPCAPS_TEXGEN_SPHEREMAP = 0x00000100;
|
|
const UINT WINED3DVTXPCAPS_NO_TEXGEN_NONLOCALVIEWER = 0x00000200;
|
|
|
|
const UINT WINED3DCURSORCAPS_COLOR = 0x00000001;
|
|
const UINT WINED3DCURSORCAPS_LOWRES = 0x00000002;
|
|
|
|
const UINT WINED3DDEVCAPS_FLOATTLVERTEX = 0x00000001;
|
|
const UINT WINED3DDEVCAPS_SORTINCREASINGZ = 0x00000002;
|
|
const UINT WINED3DDEVCAPS_SORTDECREASINGZ = 0X00000004;
|
|
const UINT WINED3DDEVCAPS_SORTEXACT = 0x00000008;
|
|
const UINT WINED3DDEVCAPS_EXECUTESYSTEMMEMORY = 0x00000010;
|
|
const UINT WINED3DDEVCAPS_EXECUTEVIDEOMEMORY = 0x00000020;
|
|
const UINT WINED3DDEVCAPS_TLVERTEXSYSTEMMEMORY = 0x00000040;
|
|
const UINT WINED3DDEVCAPS_TLVERTEXVIDEOMEMORY = 0x00000080;
|
|
const UINT WINED3DDEVCAPS_TEXTURESYSTEMMEMORY = 0x00000100;
|
|
const UINT WINED3DDEVCAPS_TEXTUREVIDEOMEMORY = 0x00000200;
|
|
const UINT WINED3DDEVCAPS_DRAWPRIMTLVERTEX = 0x00000400;
|
|
const UINT WINED3DDEVCAPS_CANRENDERAFTERFLIP = 0x00000800;
|
|
const UINT WINED3DDEVCAPS_TEXTURENONLOCALVIDMEM = 0x00001000;
|
|
const UINT WINED3DDEVCAPS_DRAWPRIMITIVES2 = 0x00002000;
|
|
const UINT WINED3DDEVCAPS_SEPARATETEXTUREMEMORIES = 0x00004000;
|
|
const UINT WINED3DDEVCAPS_DRAWPRIMITIVES2EX = 0x00008000;
|
|
const UINT WINED3DDEVCAPS_HWTRANSFORMANDLIGHT = 0x00010000;
|
|
const UINT WINED3DDEVCAPS_CANBLTSYSTONONLOCAL = 0x00020000;
|
|
const UINT WINED3DDEVCAPS_HWRASTERIZATION = 0x00080000;
|
|
const UINT WINED3DDEVCAPS_PUREDEVICE = 0x00100000;
|
|
const UINT WINED3DDEVCAPS_QUINTICRTPATCHES = 0x00200000;
|
|
const UINT WINED3DDEVCAPS_RTPATCHES = 0x00400000;
|
|
const UINT WINED3DDEVCAPS_RTPATCHHANDLEZERO = 0x00800000;
|
|
const UINT WINED3DDEVCAPS_NPATCHES = 0x01000000;
|
|
|
|
/* dwDDFX */
|
|
/* arithmetic stretching along y axis */
|
|
const UINT WINEDDBLTFX_ARITHSTRETCHY = 0x00000001;
|
|
/* mirror on y axis */
|
|
const UINT WINEDDBLTFX_MIRRORLEFTRIGHT = 0x00000002;
|
|
/* mirror on x axis */
|
|
const UINT WINEDDBLTFX_MIRRORUPDOWN = 0x00000004;
|
|
/* do not tear */
|
|
const UINT WINEDDBLTFX_NOTEARING = 0x00000008;
|
|
/* 180 degrees clockwise rotation */
|
|
const UINT WINEDDBLTFX_ROTATE180 = 0x00000010;
|
|
/* 270 degrees clockwise rotation */
|
|
const UINT WINEDDBLTFX_ROTATE270 = 0x00000020;
|
|
/* 90 degrees clockwise rotation */
|
|
const UINT WINEDDBLTFX_ROTATE90 = 0x00000040;
|
|
/* dwZBufferLow and dwZBufferHigh specify limits to the copied Z values */
|
|
const UINT WINEDDBLTFX_ZBUFFERRANGE = 0x00000080;
|
|
/* add dwZBufferBaseDest to every source z value before compare */
|
|
const UINT WINEDDBLTFX_ZBUFFERBASEDEST = 0x00000100;
|
|
|
|
/* dwFlags for Blt* */
|
|
const UINT WINEDDBLT_ALPHADEST = 0x00000001;
|
|
const UINT WINEDDBLT_ALPHADESTCONSTOVERRIDE = 0x00000002;
|
|
const UINT WINEDDBLT_ALPHADESTNEG = 0x00000004;
|
|
const UINT WINEDDBLT_ALPHADESTSURFACEOVERRIDE = 0x00000008;
|
|
const UINT WINEDDBLT_ALPHAEDGEBLEND = 0x00000010;
|
|
const UINT WINEDDBLT_ALPHASRC = 0x00000020;
|
|
const UINT WINEDDBLT_ALPHASRCCONSTOVERRIDE = 0x00000040;
|
|
const UINT WINEDDBLT_ALPHASRCNEG = 0x00000080;
|
|
const UINT WINEDDBLT_ALPHASRCSURFACEOVERRIDE = 0x00000100;
|
|
const UINT WINEDDBLT_ASYNC = 0x00000200;
|
|
const UINT WINEDDBLT_COLORFILL = 0x00000400;
|
|
const UINT WINEDDBLT_DDFX = 0x00000800;
|
|
const UINT WINEDDBLT_DDROPS = 0x00001000;
|
|
const UINT WINEDDBLT_KEYDEST = 0x00002000;
|
|
const UINT WINEDDBLT_KEYDESTOVERRIDE = 0x00004000;
|
|
const UINT WINEDDBLT_KEYSRC = 0x00008000;
|
|
const UINT WINEDDBLT_KEYSRCOVERRIDE = 0x00010000;
|
|
const UINT WINEDDBLT_ROP = 0x00020000;
|
|
const UINT WINEDDBLT_ROTATIONANGLE = 0x00040000;
|
|
const UINT WINEDDBLT_ZBUFFER = 0x00080000;
|
|
const UINT WINEDDBLT_ZBUFFERDESTCONSTOVERRIDE = 0x00100000;
|
|
const UINT WINEDDBLT_ZBUFFERDESTOVERRIDE = 0x00200000;
|
|
const UINT WINEDDBLT_ZBUFFERSRCCONSTOVERRIDE = 0x00400000;
|
|
const UINT WINEDDBLT_ZBUFFERSRCOVERRIDE = 0x00800000;
|
|
const UINT WINEDDBLT_WAIT = 0x01000000;
|
|
const UINT WINEDDBLT_DEPTHFILL = 0x02000000;
|
|
const UINT WINEDDBLT_DONOTWAIT = 0x08000000;
|
|
|
|
/* dwTrans for BltFast */
|
|
const UINT WINEDDBLTFAST_NOCOLORKEY = 0x00000000;
|
|
const UINT WINEDDBLTFAST_SRCCOLORKEY = 0x00000001;
|
|
const UINT WINEDDBLTFAST_DESTCOLORKEY = 0x00000002;
|
|
const UINT WINEDDBLTFAST_WAIT = 0x00000010;
|
|
const UINT WINEDDBLTFAST_DONOTWAIT = 0x00000020;
|
|
|
|
/* DDSURFACEDESC.dwFlags */
|
|
const UINT WINEDDSD_CAPS = 0x00000001;
|
|
const UINT WINEDDSD_HEIGHT = 0x00000002;
|
|
const UINT WINEDDSD_WIDTH = 0x00000004;
|
|
const UINT WINEDDSD_PITCH = 0x00000008;
|
|
const UINT WINEDDSD_BACKBUFFERCOUNT = 0x00000020;
|
|
const UINT WINEDDSD_ZBUFFERBITDEPTH = 0x00000040;
|
|
const UINT WINEDDSD_ALPHABITDEPTH = 0x00000080;
|
|
const UINT WINEDDSD_LPSURFACE = 0x00000800;
|
|
const UINT WINEDDSD_PIXELFORMAT = 0x00001000;
|
|
const UINT WINEDDSD_CKDESTOVERLAY = 0x00002000;
|
|
const UINT WINEDDSD_CKDESTBLT = 0x00004000;
|
|
const UINT WINEDDSD_CKSRCOVERLAY = 0x00008000;
|
|
const UINT WINEDDSD_CKSRCBLT = 0x00010000;
|
|
const UINT WINEDDSD_MIPMAPCOUNT = 0x00020000;
|
|
const UINT WINEDDSD_REFRESHRATE = 0x00040000;
|
|
const UINT WINEDDSD_LINEARSIZE = 0x00080000;
|
|
const UINT WINEDDSD_TEXTURESTAGE = 0x00100000;
|
|
const UINT WINEDDSD_FVF = 0x00200000;
|
|
const UINT WINEDDSD_SRCVBHANDLE = 0x00400000;
|
|
const UINT WINEDDSD_ALL = 0x007ff9ee;
|
|
|
|
/* Set/Get Colour Key Flags */
|
|
const UINT WINEDDCKEY_COLORSPACE = 0x00000001; /* Struct is single colour space */
|
|
const UINT WINEDDCKEY_DESTBLT = 0x00000002; /* To be used as dest for blt */
|
|
const UINT WINEDDCKEY_DESTOVERLAY = 0x00000004; /* To be used as dest for CK overlays */
|
|
const UINT WINEDDCKEY_SRCBLT = 0x00000008; /* To be used as src for blt */
|
|
const UINT WINEDDCKEY_SRCOVERLAY = 0x00000010; /* To be used as src for CK overlays */
|
|
|
|
/* dwFlags for GetBltStatus */
|
|
const UINT WINEDDGBS_CANBLT = 0x00000001;
|
|
const UINT WINEDDGBS_ISBLTDONE = 0x00000002;
|
|
|
|
/* dwFlags for GetFlipStatus */
|
|
const UINT WINEDDGFS_CANFLIP = 0x00000001;
|
|
const UINT WINEDDGFS_ISFLIPDONE = 0x00000002;
|
|
|
|
/* dwFlags for Flip */
|
|
const UINT WINEDDFLIP_WAIT = 0x00000001;
|
|
const UINT WINEDDFLIP_EVEN = 0x00000002; /* only valid for overlay */
|
|
const UINT WINEDDFLIP_ODD = 0x00000004; /* only valid for overlay */
|
|
const UINT WINEDDFLIP_NOVSYNC = 0x00000008;
|
|
const UINT WINEDDFLIP_STEREO = 0x00000010;
|
|
const UINT WINEDDFLIP_DONOTWAIT = 0x00000020;
|
|
const UINT WINEDDFLIP_INTERVAL2 = 0x02000000;
|
|
const UINT WINEDDFLIP_INTERVAL3 = 0x03000000;
|
|
const UINT WINEDDFLIP_INTERVAL4 = 0x04000000;
|
|
|
|
const UINT WINEDDOVER_ALPHADEST = 0x00000001;
|
|
const UINT WINEDDOVER_ALPHADESTCONSTOVERRIDE = 0x00000002;
|
|
const UINT WINEDDOVER_ALPHADESTNEG = 0x00000004;
|
|
const UINT WINEDDOVER_ALPHADESTSURFACEOVERRIDE = 0x00000008;
|
|
const UINT WINEDDOVER_ALPHAEDGEBLEND = 0x00000010;
|
|
const UINT WINEDDOVER_ALPHASRC = 0x00000020;
|
|
const UINT WINEDDOVER_ALPHASRCCONSTOVERRIDE = 0x00000040;
|
|
const UINT WINEDDOVER_ALPHASRCNEG = 0x00000080;
|
|
const UINT WINEDDOVER_ALPHASRCSURFACEOVERRIDE = 0x00000100;
|
|
const UINT WINEDDOVER_HIDE = 0x00000200;
|
|
const UINT WINEDDOVER_KEYDEST = 0x00000400;
|
|
const UINT WINEDDOVER_KEYDESTOVERRIDE = 0x00000800;
|
|
const UINT WINEDDOVER_KEYSRC = 0x00001000;
|
|
const UINT WINEDDOVER_KEYSRCOVERRIDE = 0x00002000;
|
|
const UINT WINEDDOVER_SHOW = 0x00004000;
|
|
const UINT WINEDDOVER_ADDDIRTYRECT = 0x00008000;
|
|
const UINT WINEDDOVER_REFRESHDIRTYRECTS = 0x00010000;
|
|
const UINT WINEDDOVER_REFRESHALL = 0x00020000;
|
|
const UINT WINEDDOVER_DDFX = 0x00080000;
|
|
const UINT WINEDDOVER_AUTOFLIP = 0x00100000;
|
|
const UINT WINEDDOVER_BOB = 0x00200000;
|
|
const UINT WINEDDOVER_OVERRIDEBOBWEAVE = 0x00400000;
|
|
const UINT WINEDDOVER_INTERLEAVED = 0x00800000;
|
|
|
|
/* DirectDraw Caps */
|
|
const UINT WINEDDSCAPS_RESERVED1 = 0x00000001;
|
|
const UINT WINEDDSCAPS_ALPHA = 0x00000002;
|
|
const UINT WINEDDSCAPS_BACKBUFFER = 0x00000004;
|
|
const UINT WINEDDSCAPS_COMPLEX = 0x00000008;
|
|
const UINT WINEDDSCAPS_FLIP = 0x00000010;
|
|
const UINT WINEDDSCAPS_FRONTBUFFER = 0x00000020;
|
|
const UINT WINEDDSCAPS_OFFSCREENPLAIN = 0x00000040;
|
|
const UINT WINEDDSCAPS_OVERLAY = 0x00000080;
|
|
const UINT WINEDDSCAPS_PALETTE = 0x00000100;
|
|
const UINT WINEDDSCAPS_PRIMARYSURFACE = 0x00000200;
|
|
const UINT WINEDDSCAPS_PRIMARYSURFACELEFT = 0x00000400;
|
|
const UINT WINEDDSCAPS_SYSTEMMEMORY = 0x00000800;
|
|
const UINT WINEDDSCAPS_TEXTURE = 0x00001000;
|
|
const UINT WINEDDSCAPS_3DDEVICE = 0x00002000;
|
|
const UINT WINEDDSCAPS_VIDEOMEMORY = 0x00004000;
|
|
const UINT WINEDDSCAPS_VISIBLE = 0x00008000;
|
|
const UINT WINEDDSCAPS_WRITEONLY = 0x00010000;
|
|
const UINT WINEDDSCAPS_ZBUFFER = 0x00020000;
|
|
const UINT WINEDDSCAPS_OWNDC = 0x00040000;
|
|
const UINT WINEDDSCAPS_LIVEVIDEO = 0x00080000;
|
|
const UINT WINEDDSCAPS_HWCODEC = 0x00100000;
|
|
const UINT WINEDDSCAPS_MODEX = 0x00200000;
|
|
const UINT WINEDDSCAPS_MIPMAP = 0x00400000;
|
|
const UINT WINEDDSCAPS_RESERVED2 = 0x00800000;
|
|
const UINT WINEDDSCAPS_ALLOCONLOAD = 0x04000000;
|
|
const UINT WINEDDSCAPS_VIDEOPORT = 0x08000000;
|
|
const UINT WINEDDSCAPS_LOCALVIDMEM = 0x10000000;
|
|
const UINT WINEDDSCAPS_NONLOCALVIDMEM = 0x20000000;
|
|
const UINT WINEDDSCAPS_STANDARDVGAMODE = 0x40000000;
|
|
const UINT WINEDDSCAPS_OPTIMIZED = 0x80000000;
|
|
|
|
const UINT WINEDDCKEYCAPS_DESTBLT = 0x00000001;
|
|
const UINT WINEDDCKEYCAPS_DESTBLTCLRSPACE = 0x00000002;
|
|
const UINT WINEDDCKEYCAPS_DESTBLTCLRSPACEYUV = 0x00000004;
|
|
const UINT WINEDDCKEYCAPS_DESTBLTYUV = 0x00000008;
|
|
const UINT WINEDDCKEYCAPS_DESTOVERLAY = 0x00000010;
|
|
const UINT WINEDDCKEYCAPS_DESTOVERLAYCLRSPACE = 0x00000020;
|
|
const UINT WINEDDCKEYCAPS_DESTOVERLAYCLRSPACEYUV = 0x00000040;
|
|
const UINT WINEDDCKEYCAPS_DESTOVERLAYONEACTIVE = 0x00000080;
|
|
const UINT WINEDDCKEYCAPS_DESTOVERLAYYUV = 0x00000100;
|
|
const UINT WINEDDCKEYCAPS_SRCBLT = 0x00000200;
|
|
const UINT WINEDDCKEYCAPS_SRCBLTCLRSPACE = 0x00000400;
|
|
const UINT WINEDDCKEYCAPS_SRCBLTCLRSPACEYUV = 0x00000800;
|
|
const UINT WINEDDCKEYCAPS_SRCBLTYUV = 0x00001000;
|
|
const UINT WINEDDCKEYCAPS_SRCOVERLAY = 0x00002000;
|
|
const UINT WINEDDCKEYCAPS_SRCOVERLAYCLRSPACE = 0x00004000;
|
|
const UINT WINEDDCKEYCAPS_SRCOVERLAYCLRSPACEYUV = 0x00008000;
|
|
const UINT WINEDDCKEYCAPS_SRCOVERLAYONEACTIVE = 0x00010000;
|
|
const UINT WINEDDCKEYCAPS_SRCOVERLAYYUV = 0x00020000;
|
|
const UINT WINEDDCKEYCAPS_NOCOSTOVERLAY = 0x00040000;
|
|
|
|
const UINT WINEDDFXCAPS_BLTALPHA = 0x00000001;
|
|
const UINT WINEDDFXCAPS_OVERLAYALPHA = 0x00000004;
|
|
const UINT WINEDDFXCAPS_BLTARITHSTRETCHYN = 0x00000010;
|
|
const UINT WINEDDFXCAPS_BLTARITHSTRETCHY = 0x00000020;
|
|
const UINT WINEDDFXCAPS_BLTMIRRORLEFTRIGHT = 0x00000040;
|
|
const UINT WINEDDFXCAPS_BLTMIRRORUPDOWN = 0x00000080;
|
|
const UINT WINEDDFXCAPS_BLTROTATION = 0x00000100;
|
|
const UINT WINEDDFXCAPS_BLTROTATION90 = 0x00000200;
|
|
const UINT WINEDDFXCAPS_BLTSHRINKX = 0x00000400;
|
|
const UINT WINEDDFXCAPS_BLTSHRINKXN = 0x00000800;
|
|
const UINT WINEDDFXCAPS_BLTSHRINKY = 0x00001000;
|
|
const UINT WINEDDFXCAPS_BLTSHRINKYN = 0x00002000;
|
|
const UINT WINEDDFXCAPS_BLTSTRETCHX = 0x00004000;
|
|
const UINT WINEDDFXCAPS_BLTSTRETCHXN = 0x00008000;
|
|
const UINT WINEDDFXCAPS_BLTSTRETCHY = 0x00010000;
|
|
const UINT WINEDDFXCAPS_BLTSTRETCHYN = 0x00020000;
|
|
const UINT WINEDDFXCAPS_OVERLAYARITHSTRETCHY = 0x00040000;
|
|
const UINT WINEDDFXCAPS_OVERLAYARITHSTRETCHYN = 0x00000008;
|
|
const UINT WINEDDFXCAPS_OVERLAYSHRINKX = 0x00080000;
|
|
const UINT WINEDDFXCAPS_OVERLAYSHRINKXN = 0x00100000;
|
|
const UINT WINEDDFXCAPS_OVERLAYSHRINKY = 0x00200000;
|
|
const UINT WINEDDFXCAPS_OVERLAYSHRINKYN = 0x00400000;
|
|
const UINT WINEDDFXCAPS_OVERLAYSTRETCHX = 0x00800000;
|
|
const UINT WINEDDFXCAPS_OVERLAYSTRETCHXN = 0x01000000;
|
|
const UINT WINEDDFXCAPS_OVERLAYSTRETCHY = 0x02000000;
|
|
const UINT WINEDDFXCAPS_OVERLAYSTRETCHYN = 0x04000000;
|
|
const UINT WINEDDFXCAPS_OVERLAYMIRRORLEFTRIGHT = 0x08000000;
|
|
const UINT WINEDDFXCAPS_OVERLAYMIRRORUPDOWN = 0x10000000;
|
|
|
|
const UINT WINEDDCAPS_3D = 0x00000001;
|
|
const UINT WINEDDCAPS_ALIGNBOUNDARYDEST = 0x00000002;
|
|
const UINT WINEDDCAPS_ALIGNSIZEDEST = 0x00000004;
|
|
const UINT WINEDDCAPS_ALIGNBOUNDARYSRC = 0x00000008;
|
|
const UINT WINEDDCAPS_ALIGNSIZESRC = 0x00000010;
|
|
const UINT WINEDDCAPS_ALIGNSTRIDE = 0x00000020;
|
|
const UINT WINEDDCAPS_BLT = 0x00000040;
|
|
const UINT WINEDDCAPS_BLTQUEUE = 0x00000080;
|
|
const UINT WINEDDCAPS_BLTFOURCC = 0x00000100;
|
|
const UINT WINEDDCAPS_BLTSTRETCH = 0x00000200;
|
|
const UINT WINEDDCAPS_GDI = 0x00000400;
|
|
const UINT WINEDDCAPS_OVERLAY = 0x00000800;
|
|
const UINT WINEDDCAPS_OVERLAYCANTCLIP = 0x00001000;
|
|
const UINT WINEDDCAPS_OVERLAYFOURCC = 0x00002000;
|
|
const UINT WINEDDCAPS_OVERLAYSTRETCH = 0x00004000;
|
|
const UINT WINEDDCAPS_PALETTE = 0x00008000;
|
|
const UINT WINEDDCAPS_PALETTEVSYNC = 0x00010000;
|
|
const UINT WINEDDCAPS_READSCANLINE = 0x00020000;
|
|
const UINT WINEDDCAPS_STEREOVIEW = 0x00040000;
|
|
const UINT WINEDDCAPS_VBI = 0x00080000;
|
|
const UINT WINEDDCAPS_ZBLTS = 0x00100000;
|
|
const UINT WINEDDCAPS_ZOVERLAYS = 0x00200000;
|
|
const UINT WINEDDCAPS_COLORKEY = 0x00400000;
|
|
const UINT WINEDDCAPS_ALPHA = 0x00800000;
|
|
const UINT WINEDDCAPS_COLORKEYHWASSIST = 0x01000000;
|
|
const UINT WINEDDCAPS_NOHARDWARE = 0x02000000;
|
|
const UINT WINEDDCAPS_BLTCOLORFILL = 0x04000000;
|
|
const UINT WINEDDCAPS_BANKSWITCHED = 0x08000000;
|
|
const UINT WINEDDCAPS_BLTDEPTHFILL = 0x10000000;
|
|
const UINT WINEDDCAPS_CANCLIP = 0x20000000;
|
|
const UINT WINEDDCAPS_CANCLIPSTRETCHED = 0x40000000;
|
|
const UINT WINEDDCAPS_CANBLTSYSMEM = 0x80000000;
|
|
|
|
const UINT WINEDDCAPS2_CERTIFIED = 0x00000001;
|
|
const UINT WINEDDCAPS2_NO2DDURING3DSCENE = 0x00000002;
|
|
const UINT WINEDDCAPS2_VIDEOPORT = 0x00000004;
|
|
const UINT WINEDDCAPS2_AUTOFLIPOVERLAY = 0x00000008;
|
|
const UINT WINEDDCAPS2_CANBOBINTERLEAVED = 0x00000010;
|
|
const UINT WINEDDCAPS2_CANBOBNONINTERLEAVED = 0x00000020;
|
|
const UINT WINEDDCAPS2_COLORCONTROLOVERLAY = 0x00000040;
|
|
const UINT WINEDDCAPS2_COLORCONTROLPRIMARY = 0x00000080;
|
|
const UINT WINEDDCAPS2_CANDROPZ16BIT = 0x00000100;
|
|
const UINT WINEDDCAPS2_NONLOCALVIDMEM = 0x00000200;
|
|
const UINT WINEDDCAPS2_NONLOCALVIDMEMCAPS = 0x00000400;
|
|
const UINT WINEDDCAPS2_NOPAGELOCKREQUIRED = 0x00000800;
|
|
const UINT WINEDDCAPS2_WIDESURFACES = 0x00001000;
|
|
const UINT WINEDDCAPS2_CANFLIPODDEVEN = 0x00002000;
|
|
const UINT WINEDDCAPS2_CANBOBHARDWARE = 0x00004000;
|
|
const UINT WINEDDCAPS2_COPYFOURCC = 0x00008000;
|
|
const UINT WINEDDCAPS2_PRIMARYGAMMA = 0x00020000;
|
|
const UINT WINEDDCAPS2_CANRENDERWINDOWED = 0x00080000;
|
|
const UINT WINEDDCAPS2_CANCALIBRATEGAMMA = 0x00100000;
|
|
const UINT WINEDDCAPS2_FLIPINTERVAL = 0x00200000;
|
|
const UINT WINEDDCAPS2_FLIPNOVSYNC = 0x00400000;
|
|
const UINT WINEDDCAPS2_CANMANAGETEXTURE = 0x00800000;
|
|
const UINT WINEDDCAPS2_TEXMANINNONLOCALVIDMEM = 0x01000000;
|
|
const UINT WINEDDCAPS2_STEREO = 0x02000000;
|
|
const UINT WINEDDCAPS2_SYSTONONLOCAL_AS_SYSTOLOCAL = 0x04000000;
|
|
|
|
/* DDCAPS.d */
|
|
const UINT WINEDDPCAPS_4BIT = 0x00000001;
|
|
const UINT WINEDDPCAPS_8BITENTRIES = 0x00000002;
|
|
const UINT WINEDDPCAPS_8BIT = 0x00000004;
|
|
const UINT WINEDDPCAPS_INITIALIZE = 0x00000008;
|
|
const UINT WINEDDPCAPS_PRIMARYSURFACE = 0x00000010;
|
|
const UINT WINEDDPCAPS_PRIMARYSURFACELEFT = 0x00000020;
|
|
const UINT WINEDDPCAPS_ALLOW256 = 0x00000040;
|
|
const UINT WINEDDPCAPS_VSYNC = 0x00000080;
|
|
const UINT WINEDDPCAPS_1BIT = 0x00000100;
|
|
const UINT WINEDDPCAPS_2BIT = 0x00000200;
|
|
const UINT WINEDDPCAPS_ALPHA = 0x00000400;
|
|
|
|
typedef struct _WINED3DDISPLAYMODE
|
|
{
|
|
UINT Width;
|
|
UINT Height;
|
|
UINT RefreshRate;
|
|
WINED3DFORMAT Format;
|
|
} WINED3DDISPLAYMODE;
|
|
|
|
typedef struct _WINED3DCOLORVALUE
|
|
{
|
|
float r;
|
|
float g;
|
|
float b;
|
|
float a;
|
|
} WINED3DCOLORVALUE;
|
|
|
|
typedef struct _WINED3DVECTOR
|
|
{
|
|
float x;
|
|
float y;
|
|
float z;
|
|
} WINED3DVECTOR;
|
|
|
|
typedef struct _WINED3DMATRIX
|
|
{
|
|
union
|
|
{
|
|
struct
|
|
{
|
|
float _11, _12, _13, _14;
|
|
float _21, _22, _23, _24;
|
|
float _31, _32, _33, _34;
|
|
float _41, _42, _43, _44;
|
|
} DUMMYSTRUCTNAME;
|
|
float m[4][4];
|
|
} DUMMYUNIONNAME;
|
|
} WINED3DMATRIX;
|
|
|
|
typedef struct _WINED3DRECT
|
|
{
|
|
LONG x1;
|
|
LONG y1;
|
|
LONG x2;
|
|
LONG y2;
|
|
} WINED3DRECT;
|
|
|
|
typedef struct _WINED3DLIGHT
|
|
{
|
|
WINED3DLIGHTTYPE Type;
|
|
WINED3DCOLORVALUE Diffuse;
|
|
WINED3DCOLORVALUE Specular;
|
|
WINED3DCOLORVALUE Ambient;
|
|
WINED3DVECTOR Position;
|
|
WINED3DVECTOR Direction;
|
|
float Range;
|
|
float Falloff;
|
|
float Attenuation0;
|
|
float Attenuation1;
|
|
float Attenuation2;
|
|
float Theta;
|
|
float Phi;
|
|
} WINED3DLIGHT;
|
|
|
|
typedef struct _WINED3DMATERIAL
|
|
{
|
|
WINED3DCOLORVALUE Diffuse;
|
|
WINED3DCOLORVALUE Ambient;
|
|
WINED3DCOLORVALUE Specular;
|
|
WINED3DCOLORVALUE Emissive;
|
|
float Power;
|
|
} WINED3DMATERIAL;
|
|
|
|
typedef struct _WINED3DVIEWPORT
|
|
{
|
|
DWORD X;
|
|
DWORD Y;
|
|
DWORD Width;
|
|
DWORD Height;
|
|
float MinZ;
|
|
float MaxZ;
|
|
} WINED3DVIEWPORT;
|
|
|
|
typedef struct _WINED3DGAMMARAMP
|
|
{
|
|
WORD red[256];
|
|
WORD green[256];
|
|
WORD blue[256];
|
|
} WINED3DGAMMARAMP;
|
|
|
|
typedef struct _WINED3DLINEPATTERN
|
|
{
|
|
WORD wRepeatFactor;
|
|
WORD wLinePattern;
|
|
} WINED3DLINEPATTERN;
|
|
|
|
typedef struct _WINEDD3DRECTPATCH_INFO
|
|
{
|
|
UINT StartVertexOffsetWidth;
|
|
UINT StartVertexOffsetHeight;
|
|
UINT Width;
|
|
UINT Height;
|
|
UINT Stride;
|
|
WINED3DBASISTYPE Basis;
|
|
WINED3DDEGREETYPE Degree;
|
|
} WINED3DRECTPATCH_INFO;
|
|
|
|
typedef struct _WINED3DTRIPATCH_INFO
|
|
{
|
|
UINT StartVertexOffset;
|
|
UINT NumVertices;
|
|
WINED3DBASISTYPE Basis;
|
|
WINED3DDEGREETYPE Degree;
|
|
} WINED3DTRIPATCH_INFO;
|
|
|
|
typedef struct _WINED3DADAPTER_IDENTIFIER
|
|
{
|
|
char *Driver;
|
|
char *Description;
|
|
char *DeviceName;
|
|
LARGE_INTEGER *DriverVersion;
|
|
DWORD *VendorId;
|
|
DWORD *DeviceId;
|
|
DWORD *SubSysId;
|
|
DWORD *Revision;
|
|
GUID *DeviceIdentifier;
|
|
DWORD *WHQLLevel;
|
|
} WINED3DADAPTER_IDENTIFIER;
|
|
|
|
typedef struct _WINED3DPRESENT_PARAMETERS
|
|
{
|
|
UINT BackBufferWidth;
|
|
UINT BackBufferHeight;
|
|
WINED3DFORMAT BackBufferFormat;
|
|
UINT BackBufferCount;
|
|
WINED3DMULTISAMPLE_TYPE MultiSampleType;
|
|
DWORD MultiSampleQuality;
|
|
WINED3DSWAPEFFECT SwapEffect;
|
|
HWND hDeviceWindow;
|
|
BOOL Windowed;
|
|
BOOL EnableAutoDepthStencil;
|
|
WINED3DFORMAT AutoDepthStencilFormat;
|
|
DWORD Flags;
|
|
UINT FullScreen_RefreshRateInHz;
|
|
UINT PresentationInterval;
|
|
BOOL AutoRestoreDisplayMode;
|
|
} WINED3DPRESENT_PARAMETERS;
|
|
|
|
typedef struct _WINED3DSURFACE_DESC
|
|
{
|
|
WINED3DFORMAT *Format;
|
|
WINED3DRESOURCETYPE *Type;
|
|
DWORD *Usage;
|
|
WINED3DPOOL *Pool;
|
|
UINT *Size;
|
|
WINED3DMULTISAMPLE_TYPE *MultiSampleType;
|
|
DWORD *MultiSampleQuality;
|
|
UINT *Width;
|
|
UINT *Height;
|
|
} WINED3DSURFACE_DESC;
|
|
|
|
typedef struct _WINED3DVOLUME_DESC
|
|
{
|
|
WINED3DFORMAT *Format;
|
|
WINED3DRESOURCETYPE *Type;
|
|
DWORD *Usage;
|
|
WINED3DPOOL *Pool;
|
|
UINT *Size;
|
|
UINT *Width;
|
|
UINT *Height;
|
|
UINT *Depth;
|
|
} WINED3DVOLUME_DESC;
|
|
|
|
typedef struct _WINED3DCLIPSTATUS
|
|
{
|
|
DWORD ClipUnion;
|
|
DWORD ClipIntersection;
|
|
} WINED3DCLIPSTATUS;
|
|
|
|
typedef struct _WINED3DVERTEXELEMENT
|
|
{
|
|
WORD Stream;
|
|
WORD Offset;
|
|
BYTE Type;
|
|
BYTE Method;
|
|
BYTE Usage;
|
|
BYTE UsageIndex;
|
|
int Reg; /* DirectX 8 */
|
|
} WINED3DVERTEXELEMENT, *LPWINED3DVERTEXELEMENT;
|
|
|
|
typedef struct _WINED3DDEVICE_CREATION_PARAMETERS
|
|
{
|
|
UINT AdapterOrdinal;
|
|
WINED3DDEVTYPE DeviceType;
|
|
HWND hFocusWindow;
|
|
DWORD BehaviorFlags;
|
|
} WINED3DDEVICE_CREATION_PARAMETERS;
|
|
|
|
typedef struct _WINED3DDEVINFO_BANDWIDTHTIMINGS
|
|
{
|
|
float MaxBandwidthUtilized;
|
|
float FrontEndUploadMemoryUtilizedPercent;
|
|
float VertexRateUtilizedPercent;
|
|
float TriangleSetupRateUtilizedPercent;
|
|
float FillRateUtilizedPercent;
|
|
} WINED3DDEVINFO_BANDWIDTHTIMINGS;
|
|
|
|
typedef struct _WINED3DDEVINFO_CACHEUTILIZATION
|
|
{
|
|
float TextureCacheHitRate;
|
|
float PostTransformVertexCacheHitRate;
|
|
} WINED3DDEVINFO_CACHEUTILIZATION;
|
|
|
|
typedef struct _WINED3DDEVINFO_INTERFACETIMINGS
|
|
{
|
|
float WaitingForGPUToUseApplicationResourceTimePercent;
|
|
float WaitingForGPUToAcceptMoreCommandsTimePercent;
|
|
float WaitingForGPUToStayWithinLatencyTimePercent;
|
|
float WaitingForGPUExclusiveResourceTimePercent;
|
|
float WaitingForGPUOtherTimePercent;
|
|
} WINED3DDEVINFO_INTERFACETIMINGS;
|
|
|
|
typedef struct _WINED3DDEVINFO_PIPELINETIMINGS
|
|
{
|
|
float VertexProcessingTimePercent;
|
|
float PixelProcessingTimePercent;
|
|
float OtherGPUProcessingTimePercent;
|
|
float GPUIdleTimePercent;
|
|
} WINED3DDEVINFO_PIPELINETIMINGS;
|
|
|
|
typedef struct _WINED3DDEVINFO_STAGETIMINGS
|
|
{
|
|
float MemoryProcessingPercent;
|
|
float ComputationProcessingPercent;
|
|
} WINED3DDEVINFO_STAGETIMINGS;
|
|
|
|
typedef struct _WINED3DRASTER_STATUS
|
|
{
|
|
BOOL InVBlank;
|
|
UINT ScanLine;
|
|
} WINED3DRASTER_STATUS;
|
|
|
|
typedef struct WINED3DRESOURCESTATS
|
|
{
|
|
BOOL bThrashing;
|
|
DWORD ApproxBytesDownloaded;
|
|
DWORD NumEvicts;
|
|
DWORD NumVidCreates;
|
|
DWORD LastPri;
|
|
DWORD NumUsed;
|
|
DWORD NumUsedInVidMem;
|
|
DWORD WorkingSet;
|
|
DWORD WorkingSetBytes;
|
|
DWORD TotalManaged;
|
|
DWORD TotalBytes;
|
|
} WINED3DRESOURCESTATS;
|
|
|
|
typedef struct _WINED3DDEVINFO_RESOURCEMANAGER
|
|
{
|
|
WINED3DRESOURCESTATS stats[WINED3DRTYPECOUNT];
|
|
} WINED3DDEVINFO_RESOURCEMANAGER;
|
|
|
|
typedef struct _WINED3DDEVINFO_VERTEXSTATS
|
|
{
|
|
DWORD NumRenderedTriangles;
|
|
DWORD NumExtraClippingTriangles;
|
|
} WINED3DDEVINFO_VERTEXSTATS;
|
|
|
|
typedef struct _WINED3DLOCKED_RECT
|
|
{
|
|
INT Pitch;
|
|
void *pBits;
|
|
} WINED3DLOCKED_RECT;
|
|
|
|
typedef struct _WINED3DLOCKED_BOX
|
|
{
|
|
INT RowPitch;
|
|
INT SlicePitch;
|
|
void *pBits;
|
|
} WINED3DLOCKED_BOX;
|
|
|
|
typedef struct _WINED3DBOX
|
|
{
|
|
UINT Left;
|
|
UINT Top;
|
|
UINT Right;
|
|
UINT Bottom;
|
|
UINT Front;
|
|
UINT Back;
|
|
} WINED3DBOX;
|
|
|
|
/*Vertex cache optimization hints.*/
|
|
typedef struct WINED3DDEVINFO_VCACHE
|
|
{
|
|
DWORD Pattern; /* Must be a 4 char code FOURCC (e.g. CACH) */
|
|
DWORD OptMethod; /* 0 to get the longest strips, 1 vertex cache */
|
|
DWORD CacheSize; /* Cache size to use (only valid if OptMethod==1) */
|
|
DWORD MagicNumber; /* Internal for deciding when to restart strips,
|
|
non user modifiable (only valid if OptMethod==1) */
|
|
} WINED3DDEVINFO_VCACHE;
|
|
|
|
typedef struct _WINED3DVERTEXBUFFER_DESC
|
|
{
|
|
WINED3DFORMAT Format;
|
|
WINED3DRESOURCETYPE Type;
|
|
DWORD Usage;
|
|
WINED3DPOOL Pool;
|
|
UINT Size;
|
|
DWORD FVF;
|
|
} WINED3DVERTEXBUFFER_DESC;
|
|
|
|
typedef struct _WINED3DINDEXBUFFER_DESC
|
|
{
|
|
WINED3DFORMAT Format;
|
|
WINED3DRESOURCETYPE Type;
|
|
DWORD Usage;
|
|
WINED3DPOOL Pool;
|
|
UINT Size;
|
|
} WINED3DINDEXBUFFER_DESC;
|
|
|
|
typedef struct glDescriptor
|
|
{
|
|
UINT textureName;
|
|
int level;
|
|
int /*GLenum*/ target;
|
|
int /*GLenum*/ glFormat;
|
|
int /*GLenum*/ glFormatInternal;
|
|
int /*GLenum*/ glType;
|
|
} glDescriptor;
|
|
|
|
typedef struct WineDirect3DStridedData
|
|
{
|
|
const BYTE *lpData; /* Pointer to start of data */
|
|
DWORD dwStride; /* Stride between occurrences of this data */
|
|
DWORD dwType; /* Type (as in D3DVSDT_TYPE) */
|
|
int VBO; /* Vertex buffer object this data is in */
|
|
UINT streamNo; /* D3D stream number */
|
|
} WineDirect3DStridedData;
|
|
|
|
typedef struct WineDirect3DVertexStridedData
|
|
{
|
|
/*
|
|
* IMPORTANT:
|
|
* This structure can be accessed in two ways: Named access, and array
|
|
* access. Please note that named access is only valid with the fixed
|
|
* function vertex pipeline, and the arrays are only valid with the
|
|
* programmable vertex pipeline(vertex shaders).
|
|
*/
|
|
union
|
|
{
|
|
struct
|
|
{
|
|
/* Do not add or reorder fields here,
|
|
* so this can be indexed as an array */
|
|
WineDirect3DStridedData position;
|
|
WineDirect3DStridedData blendWeights;
|
|
WineDirect3DStridedData blendMatrixIndices;
|
|
WineDirect3DStridedData normal;
|
|
WineDirect3DStridedData pSize;
|
|
WineDirect3DStridedData diffuse;
|
|
WineDirect3DStridedData specular;
|
|
WineDirect3DStridedData texCoords[WINED3DDP_MAXTEXCOORD];
|
|
WineDirect3DStridedData position2; /* tween data */
|
|
WineDirect3DStridedData normal2; /* tween data */
|
|
WineDirect3DStridedData tangent;
|
|
WineDirect3DStridedData binormal;
|
|
WineDirect3DStridedData tessFactor;
|
|
WineDirect3DStridedData fog;
|
|
WineDirect3DStridedData depth;
|
|
WineDirect3DStridedData sample;
|
|
|
|
/* Add fields here */
|
|
} s;
|
|
WineDirect3DStridedData input[16]; /* Indexed by constants in D3DVSDE_REGISTER */
|
|
} u;
|
|
|
|
BOOL position_transformed;
|
|
WORD swizzle_map; /* MAX_ATTRIBS, 16 */
|
|
WORD use_map; /* MAX_ATTRIBS, 16 */
|
|
} WineDirect3DVertexStridedData;
|
|
|
|
typedef struct _WINED3DVSHADERCAPS2_0
|
|
{
|
|
DWORD Caps;
|
|
INT DynamicFlowControlDepth;
|
|
INT NumTemps;
|
|
INT StaticFlowControlDepth;
|
|
} WINED3DVSHADERCAPS2_0;
|
|
|
|
typedef struct _WINED3DPSHADERCAPS2_0
|
|
{
|
|
DWORD Caps;
|
|
INT DynamicFlowControlDepth;
|
|
INT NumTemps;
|
|
INT StaticFlowControlDepth;
|
|
INT NumInstructionSlots;
|
|
} WINED3DPSHADERCAPS2_0;
|
|
|
|
typedef struct _WINEDDCAPS
|
|
{
|
|
DWORD Caps;
|
|
DWORD Caps2;
|
|
DWORD CKeyCaps;
|
|
DWORD FXCaps;
|
|
DWORD FXAlphaCaps;
|
|
DWORD PalCaps;
|
|
DWORD SVCaps;
|
|
DWORD SVBCaps;
|
|
DWORD SVBCKeyCaps;
|
|
DWORD SVBFXCaps;
|
|
DWORD VSBCaps;
|
|
DWORD VSBCKeyCaps;
|
|
DWORD VSBFXCaps;
|
|
DWORD SSBCaps;
|
|
DWORD SSBCKeyCaps;
|
|
DWORD SSBFXCaps;
|
|
DWORD ddsCaps;
|
|
DWORD StrideAlign;
|
|
} WINEDDCAPS;
|
|
|
|
typedef struct _WINED3DCAPS
|
|
{
|
|
WINED3DDEVTYPE DeviceType;
|
|
UINT AdapterOrdinal;
|
|
|
|
DWORD Caps;
|
|
DWORD Caps2;
|
|
DWORD Caps3;
|
|
DWORD PresentationIntervals;
|
|
|
|
DWORD CursorCaps;
|
|
DWORD DevCaps;
|
|
DWORD PrimitiveMiscCaps;
|
|
DWORD RasterCaps;
|
|
DWORD ZCmpCaps;
|
|
DWORD SrcBlendCaps;
|
|
DWORD DestBlendCaps;
|
|
DWORD AlphaCmpCaps;
|
|
DWORD ShadeCaps;
|
|
DWORD TextureCaps;
|
|
DWORD TextureFilterCaps;
|
|
DWORD CubeTextureFilterCaps;
|
|
DWORD VolumeTextureFilterCaps;
|
|
DWORD TextureAddressCaps;
|
|
DWORD VolumeTextureAddressCaps;
|
|
DWORD LineCaps;
|
|
|
|
DWORD MaxTextureWidth;
|
|
DWORD MaxTextureHeight;
|
|
DWORD MaxVolumeExtent;
|
|
DWORD MaxTextureRepeat;
|
|
DWORD MaxTextureAspectRatio;
|
|
DWORD MaxAnisotropy;
|
|
float MaxVertexW;
|
|
|
|
float GuardBandLeft;
|
|
float GuardBandTop;
|
|
float GuardBandRight;
|
|
float GuardBandBottom;
|
|
|
|
float ExtentsAdjust;
|
|
DWORD StencilCaps;
|
|
|
|
DWORD FVFCaps;
|
|
DWORD TextureOpCaps;
|
|
DWORD MaxTextureBlendStages;
|
|
DWORD MaxSimultaneousTextures;
|
|
|
|
DWORD VertexProcessingCaps;
|
|
DWORD MaxActiveLights;
|
|
DWORD MaxUserClipPlanes;
|
|
DWORD MaxVertexBlendMatrices;
|
|
DWORD MaxVertexBlendMatrixIndex;
|
|
|
|
float MaxPointSize;
|
|
|
|
DWORD MaxPrimitiveCount;
|
|
DWORD MaxVertexIndex;
|
|
DWORD MaxStreams;
|
|
DWORD MaxStreamStride;
|
|
|
|
DWORD VertexShaderVersion;
|
|
DWORD MaxVertexShaderConst;
|
|
|
|
DWORD PixelShaderVersion;
|
|
float PixelShader1xMaxValue;
|
|
|
|
/* DX 9 */
|
|
DWORD DevCaps2;
|
|
|
|
float MaxNpatchTessellationLevel;
|
|
DWORD Reserved5; /* undocumented */
|
|
|
|
UINT MasterAdapterOrdinal;
|
|
UINT AdapterOrdinalInGroup;
|
|
UINT NumberOfAdaptersInGroup;
|
|
DWORD DeclTypes;
|
|
DWORD NumSimultaneousRTs;
|
|
DWORD StretchRectFilterCaps;
|
|
WINED3DVSHADERCAPS2_0 VS20Caps;
|
|
WINED3DPSHADERCAPS2_0 PS20Caps;
|
|
DWORD VertexTextureFilterCaps;
|
|
DWORD MaxVShaderInstructionsExecuted;
|
|
DWORD MaxPShaderInstructionsExecuted;
|
|
DWORD MaxVertexShader30InstructionSlots;
|
|
DWORD MaxPixelShader30InstructionSlots;
|
|
DWORD Reserved2; /* Not in the microsoft headers but documented */
|
|
DWORD Reserved3;
|
|
|
|
WINEDDCAPS DirectDrawCaps;
|
|
} WINED3DCAPS;
|
|
|
|
/* DirectDraw types */
|
|
|
|
typedef struct _WINEDDCOLORKEY
|
|
{
|
|
DWORD dwColorSpaceLowValue; /* low boundary of color space that is to
|
|
* be treated as Color Key, inclusive */
|
|
DWORD dwColorSpaceHighValue; /* high boundary of color space that is
|
|
* to be treated as Color Key, inclusive */
|
|
} WINEDDCOLORKEY,*LPWINEDDCOLORKEY;
|
|
|
|
typedef struct _WINEDDBLTFX
|
|
{
|
|
DWORD dwSize; /* size of structure */
|
|
DWORD dwDDFX; /* FX operations */
|
|
DWORD dwROP; /* Win32 raster operations */
|
|
DWORD dwDDROP; /* Raster operations new for DirectDraw */
|
|
DWORD dwRotationAngle; /* Rotation angle for blt */
|
|
DWORD dwZBufferOpCode; /* ZBuffer compares */
|
|
DWORD dwZBufferLow; /* Low limit of Z buffer */
|
|
DWORD dwZBufferHigh; /* High limit of Z buffer */
|
|
DWORD dwZBufferBaseDest; /* Destination base value */
|
|
DWORD dwZDestConstBitDepth; /* Bit depth used to specify Z constant for destination */
|
|
union
|
|
{
|
|
DWORD dwZDestConst; /* Constant to use as Z buffer for dest */
|
|
struct IWineD3DSurface *lpDDSZBufferDest; /* Surface to use as Z buffer for dest */
|
|
} DUMMYUNIONNAME1;
|
|
DWORD dwZSrcConstBitDepth; /* Bit depth used to specify Z constant for source */
|
|
union
|
|
{
|
|
DWORD dwZSrcConst; /* Constant to use as Z buffer for src */
|
|
struct IWineD3DSurface *lpDDSZBufferSrc; /* Surface to use as Z buffer for src */
|
|
} DUMMYUNIONNAME2;
|
|
DWORD dwAlphaEdgeBlendBitDepth; /* Bit depth used to specify constant for alpha edge blend */
|
|
DWORD dwAlphaEdgeBlend; /* Alpha for edge blending */
|
|
DWORD dwReserved;
|
|
DWORD dwAlphaDestConstBitDepth; /* Bit depth used to specify alpha constant for destination */
|
|
union
|
|
{
|
|
DWORD dwAlphaDestConst; /* Constant to use as Alpha Channel */
|
|
struct IWineD3DSurface *lpDDSAlphaDest; /* Surface to use as Alpha Channel */
|
|
} DUMMYUNIONNAME3;
|
|
DWORD dwAlphaSrcConstBitDepth; /* Bit depth used to specify alpha constant for source */
|
|
union
|
|
{
|
|
DWORD dwAlphaSrcConst; /* Constant to use as Alpha Channel */
|
|
struct IWineD3DSurface *lpDDSAlphaSrc; /* Surface to use as Alpha Channel */
|
|
} DUMMYUNIONNAME4;
|
|
union
|
|
{
|
|
DWORD dwFillColor; /* color in RGB or Palettized */
|
|
DWORD dwFillDepth; /* depth value for z-buffer */
|
|
DWORD dwFillPixel; /* pixel val for RGBA or RGBZ */
|
|
struct IWineD3DSurface *lpDDSPattern; /* Surface to use as pattern */
|
|
} DUMMYUNIONNAME5;
|
|
WINEDDCOLORKEY ddckDestColorkey; /* DestColorkey override */
|
|
WINEDDCOLORKEY ddckSrcColorkey; /* SrcColorkey override */
|
|
} WINEDDBLTFX,*LPWINEDDBLTFX;
|
|
|
|
typedef struct _WINEDDOVERLAYFX
|
|
{
|
|
DWORD dwSize; /* size of structure */
|
|
DWORD dwAlphaEdgeBlendBitDepth; /* Bit depth used to specify constant for alpha edge blend */
|
|
DWORD dwAlphaEdgeBlend; /* Constant to use as alpha for edge blend */
|
|
DWORD dwReserved;
|
|
DWORD dwAlphaDestConstBitDepth; /* Bit depth used to specify alpha constant for destination */
|
|
union
|
|
{
|
|
DWORD dwAlphaDestConst; /* Constant to use as alpha channel for dest */
|
|
struct IWineD3DSurface *lpDDSAlphaDest; /* Surface to use as alpha channel for dest */
|
|
} DUMMYUNIONNAME1;
|
|
DWORD dwAlphaSrcConstBitDepth; /* Bit depth used to specify alpha constant for source */
|
|
union
|
|
{
|
|
DWORD dwAlphaSrcConst; /* Constant to use as alpha channel for src */
|
|
struct IWineD3DSurface *lpDDSAlphaSrc; /* Surface to use as alpha channel for src */
|
|
} DUMMYUNIONNAME2;
|
|
WINEDDCOLORKEY dckDestColorkey; /* DestColorkey override */
|
|
WINEDDCOLORKEY dckSrcColorkey; /* SrcColorkey override */
|
|
DWORD dwDDFX; /* Overlay FX */
|
|
DWORD dwFlags; /* flags */
|
|
} WINEDDOVERLAYFX;
|
|
|
|
interface IWineD3DResource;
|
|
interface IWineD3DSurface;
|
|
interface IWineD3DVolume;
|
|
interface IWineD3DSwapChain;
|
|
interface IWineD3DDevice;
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(aeb62dfc-bdcb-4f02-9519-1eeea00c15cd)
|
|
]
|
|
interface IWineD3DDeviceParent : IUnknown
|
|
{
|
|
HRESULT CreateSurface(
|
|
[in] IUnknown *superior,
|
|
[in] UINT width,
|
|
[in] UINT height,
|
|
[in] WINED3DFORMAT format,
|
|
[in] DWORD usage,
|
|
[in] WINED3DPOOL pool,
|
|
[in] UINT level,
|
|
[in] WINED3DCUBEMAP_FACES face,
|
|
[out] IWineD3DSurface **surface
|
|
);
|
|
|
|
HRESULT CreateRenderTarget(
|
|
[in] IUnknown *superior,
|
|
[in] UINT width,
|
|
[in] UINT height,
|
|
[in] WINED3DFORMAT format,
|
|
[in] WINED3DMULTISAMPLE_TYPE multisample_type,
|
|
[in] DWORD multisample_quality,
|
|
[in] BOOL lockable,
|
|
[out] IWineD3DSurface **surface
|
|
);
|
|
|
|
HRESULT CreateDepthStencilSurface(
|
|
[in] IUnknown *superior,
|
|
[in] UINT width,
|
|
[in] UINT height,
|
|
[in] WINED3DFORMAT format,
|
|
[in] WINED3DMULTISAMPLE_TYPE multisample_type,
|
|
[in] DWORD multisample_quality,
|
|
[in] BOOL discard,
|
|
[out] IWineD3DSurface **surface
|
|
);
|
|
|
|
HRESULT CreateVolume(
|
|
[in] IUnknown *superior,
|
|
[in] UINT width,
|
|
[in] UINT height,
|
|
[in] UINT depth,
|
|
[in] WINED3DFORMAT format,
|
|
[in] WINED3DPOOL pool,
|
|
[in] DWORD usage,
|
|
[out] IWineD3DVolume **volume
|
|
);
|
|
|
|
HRESULT CreateSwapChain(
|
|
[in, out] WINED3DPRESENT_PARAMETERS *present_parameters,
|
|
[out] IWineD3DSwapChain **swapchain
|
|
);
|
|
}
|
|
typedef ULONG (*D3DCB_DESTROYSURFACEFN)(IWineD3DSurface *pSurface);
|
|
typedef ULONG (*D3DCB_DESTROYVOLUMEFN)(IWineD3DVolume *pVolume);
|
|
typedef ULONG (*D3DCB_DESTROYSWAPCHAINFN)(IWineD3DSwapChain *pSwapChain);
|
|
typedef HRESULT (*D3DCB_ENUMRESOURCES)(IWineD3DResource *resource, void *pData);
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(46799311-8e0e-40ce-b2ec-ddb99f18fcb4)
|
|
]
|
|
interface IWineD3DBase : IUnknown
|
|
{
|
|
HRESULT GetParent(
|
|
[out] IUnknown **parent
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(108f9c44-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3D : IWineD3DBase
|
|
{
|
|
UINT GetAdapterCount(
|
|
);
|
|
HRESULT RegisterSoftwareDevice(
|
|
[in] void *pInitializeFunction
|
|
);
|
|
HMONITOR GetAdapterMonitor(
|
|
[in] UINT adapter_idx
|
|
);
|
|
UINT GetAdapterModeCount(
|
|
[in] UINT adapter_idx,
|
|
[in] WINED3DFORMAT format
|
|
);
|
|
HRESULT EnumAdapterModes(
|
|
[in] UINT adapter_idx,
|
|
[in] UINT mode_idx,
|
|
[in] WINED3DFORMAT format,
|
|
[out] WINED3DDISPLAYMODE *mode
|
|
);
|
|
HRESULT GetAdapterDisplayMode(
|
|
[in] UINT adapter_idx,
|
|
[out] WINED3DDISPLAYMODE *mode
|
|
);
|
|
HRESULT GetAdapterIdentifier(
|
|
[in] UINT adapter_idx,
|
|
[in] DWORD flags,
|
|
[out] WINED3DADAPTER_IDENTIFIER *identifier
|
|
);
|
|
HRESULT CheckDeviceMultiSampleType(
|
|
[in] UINT adapter_idx,
|
|
[in] WINED3DDEVTYPE device_type,
|
|
[in] WINED3DFORMAT surface_format,
|
|
[in] BOOL windowed,
|
|
[in] WINED3DMULTISAMPLE_TYPE multisample_type,
|
|
[out] DWORD *quality_levels
|
|
);
|
|
HRESULT CheckDepthStencilMatch(
|
|
[in] UINT adapter_idx,
|
|
[in] WINED3DDEVTYPE device_type,
|
|
[in] WINED3DFORMAT adapter_format,
|
|
[in] WINED3DFORMAT render_target_format,
|
|
[in] WINED3DFORMAT depth_stencil_format
|
|
);
|
|
HRESULT CheckDeviceType(
|
|
[in] UINT adapter_idx,
|
|
[in] WINED3DDEVTYPE device_type,
|
|
[in] WINED3DFORMAT display_format,
|
|
[in] WINED3DFORMAT backbuffer_format,
|
|
[in] BOOL windowed
|
|
);
|
|
HRESULT CheckDeviceFormat(
|
|
[in] UINT adaper_idx,
|
|
[in] WINED3DDEVTYPE device_type,
|
|
[in] WINED3DFORMAT adapter_format,
|
|
[in] DWORD usage,
|
|
[in] WINED3DRESOURCETYPE resource_type,
|
|
[in] WINED3DFORMAT check_format,
|
|
[in] WINED3DSURFTYPE surface_type
|
|
);
|
|
HRESULT CheckDeviceFormatConversion(
|
|
[in] UINT adapter_idx,
|
|
[in] WINED3DDEVTYPE device_type,
|
|
[in] WINED3DFORMAT source_format,
|
|
[in] WINED3DFORMAT target_format
|
|
);
|
|
HRESULT GetDeviceCaps(
|
|
[in] UINT adapter_idx,
|
|
[in] WINED3DDEVTYPE device_type,
|
|
[out] WINED3DCAPS *caps
|
|
);
|
|
HRESULT CreateDevice(
|
|
[in] UINT adapter_idx,
|
|
[in] WINED3DDEVTYPE device_type,
|
|
[in] HWND focus_window,
|
|
[in] DWORD behaviour_flags,
|
|
[in] IUnknown *parent,
|
|
[in] IWineD3DDeviceParent *device_parent,
|
|
[out] IWineD3DDevice **device
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(1f3bfb34-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DResource : IWineD3DBase
|
|
{
|
|
HRESULT GetDevice(
|
|
[out] IWineD3DDevice **device
|
|
);
|
|
HRESULT SetPrivateData(
|
|
[in] REFGUID guid,
|
|
[in] const void *data,
|
|
[in] DWORD data_size,
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT GetPrivateData(
|
|
[in] REFGUID guid,
|
|
[out] void *data,
|
|
[in, out] DWORD *data_size
|
|
);
|
|
HRESULT FreePrivateData(
|
|
[in] REFGUID guid
|
|
);
|
|
DWORD SetPriority(
|
|
[in] DWORD new_priority
|
|
);
|
|
DWORD GetPriority(
|
|
);
|
|
void PreLoad(
|
|
);
|
|
void UnLoad(
|
|
);
|
|
WINED3DRESOURCETYPE GetType(
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(217f671e-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DVertexBuffer : IWineD3DResource
|
|
{
|
|
HRESULT Lock(
|
|
[in] UINT offset,
|
|
[in] UINT size,
|
|
[out] BYTE **data,
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT Unlock(
|
|
);
|
|
HRESULT GetDesc(
|
|
[out] WINED3DVERTEXBUFFER_DESC *desc
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(3a02a54e-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DIndexBuffer : IWineD3DResource
|
|
{
|
|
HRESULT Lock(
|
|
[in] UINT offset,
|
|
[in] UINT size,
|
|
[out] BYTE **data,
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT Unlock(
|
|
);
|
|
HRESULT GetDesc(
|
|
[out] WINED3DINDEXBUFFER_DESC *desc
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(f756720c-32b9-4439-b5a3-1d6c97037d9e)
|
|
]
|
|
interface IWineD3DPalette : IWineD3DBase
|
|
{
|
|
HRESULT GetEntries(
|
|
[in] DWORD flags,
|
|
[in] DWORD start,
|
|
[in] DWORD count,
|
|
[out] PALETTEENTRY *entries
|
|
);
|
|
HRESULT GetCaps(
|
|
[out] DWORD *caps
|
|
);
|
|
HRESULT SetEntries(
|
|
[in] DWORD flags,
|
|
[in] DWORD start,
|
|
[in] DWORD count,
|
|
[in] const PALETTEENTRY *entries
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(8f2bceb1-d338-488c-ab7f-0ec980bf5d2d)
|
|
]
|
|
interface IWineD3DClipper : IWineD3DBase
|
|
{
|
|
HRESULT GetClipList(
|
|
[in] const RECT *rect,
|
|
[out] RGNDATA *clip_list,
|
|
[in, out] DWORD *clip_list_size
|
|
);
|
|
HRESULT GetHWnd(
|
|
[out] HWND *hwnd
|
|
);
|
|
HRESULT IsClipListChanged(
|
|
[out] BOOL *changed
|
|
);
|
|
HRESULT SetClipList(
|
|
[in] const RGNDATA *clip_list,
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT SetHWnd(
|
|
[in] DWORD flags,
|
|
[in] HWND hwnd
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(37cd5526-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DSurface : IWineD3DResource
|
|
{
|
|
HRESULT GetContainer(
|
|
[in] REFIID riid,
|
|
[out] void **container
|
|
);
|
|
HRESULT GetDesc(
|
|
[out] WINED3DSURFACE_DESC *desc
|
|
);
|
|
HRESULT LockRect(
|
|
[out] WINED3DLOCKED_RECT *locked_rect,
|
|
[in] const RECT *rect,
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT UnlockRect(
|
|
);
|
|
HRESULT GetDC(
|
|
[out] HDC *dc
|
|
);
|
|
HRESULT ReleaseDC(
|
|
[in] HDC dc
|
|
);
|
|
HRESULT Flip(
|
|
[in] IWineD3DSurface *override,
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT Blt(
|
|
[in] const RECT *dst_rect,
|
|
[in] IWineD3DSurface *src_surface,
|
|
[in] const RECT *src_rect,
|
|
[in] DWORD flags,
|
|
[in] const WINEDDBLTFX *blt_fx,
|
|
[in] WINED3DTEXTUREFILTERTYPE filter
|
|
);
|
|
HRESULT GetBltStatus(
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT GetFlipStatus(
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT IsLost(
|
|
);
|
|
HRESULT Restore(
|
|
);
|
|
HRESULT BltFast(
|
|
[in] DWORD dst_x,
|
|
[in] DWORD dst_y,
|
|
[in] IWineD3DSurface *src_surface,
|
|
[in] const RECT *src_rect,
|
|
[in] DWORD trans
|
|
);
|
|
HRESULT GetPalette(
|
|
[out] IWineD3DPalette **palette
|
|
);
|
|
HRESULT SetPalette(
|
|
[in] IWineD3DPalette *palette
|
|
);
|
|
HRESULT RealizePalette(
|
|
);
|
|
HRESULT SetColorKey(
|
|
[in] DWORD flags,
|
|
[in] const WINEDDCOLORKEY *color_key
|
|
);
|
|
DWORD GetPitch(
|
|
);
|
|
HRESULT SetMem(
|
|
[in] void *mem
|
|
);
|
|
HRESULT SetOverlayPosition(
|
|
[in] LONG x,
|
|
[in] LONG y
|
|
);
|
|
HRESULT GetOverlayPosition(
|
|
[out] LONG *x,
|
|
[out] LONG *y
|
|
);
|
|
HRESULT UpdateOverlayZOrder(
|
|
[in] DWORD flags,
|
|
[in] IWineD3DSurface *ref
|
|
);
|
|
HRESULT UpdateOverlay(
|
|
[in] const RECT *src_rect,
|
|
[in] IWineD3DSurface *dst_surface,
|
|
[in] const RECT *dst_rect,
|
|
[in] DWORD flags,
|
|
[in] const WINEDDOVERLAYFX *fx
|
|
);
|
|
HRESULT SetClipper(
|
|
[in] IWineD3DClipper *clipper
|
|
);
|
|
HRESULT GetClipper(
|
|
[out] IWineD3DClipper **clipper
|
|
);
|
|
HRESULT LoadTexture(
|
|
[in] BOOL srgb_mode
|
|
);
|
|
void BindTexture(
|
|
);
|
|
HRESULT SaveSnapshot(
|
|
[in] const char *filename
|
|
);
|
|
HRESULT SetContainer(
|
|
[in] IWineD3DBase *container
|
|
);
|
|
void GetGlDesc(
|
|
[out] glDescriptor **desc
|
|
);
|
|
const void *GetData(
|
|
);
|
|
HRESULT SetFormat(
|
|
[in] WINED3DFORMAT format
|
|
);
|
|
HRESULT PrivateSetup(
|
|
);
|
|
void ModifyLocation(
|
|
[in] DWORD location,
|
|
[in] BOOL persistent
|
|
);
|
|
HRESULT LoadLocation(
|
|
[in] DWORD location,
|
|
[in] const RECT *rect
|
|
);
|
|
WINED3DSURFTYPE GetImplType(
|
|
);
|
|
HRESULT DrawOverlay(
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(24769ed8-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DVolume : IWineD3DResource
|
|
{
|
|
HRESULT GetContainer(
|
|
[in] REFIID riid,
|
|
[out] void **container
|
|
);
|
|
HRESULT GetDesc(
|
|
[out] WINED3DVOLUME_DESC *desc
|
|
);
|
|
HRESULT LockBox(
|
|
[out] WINED3DLOCKED_BOX *locked_box,
|
|
[in] const WINED3DBOX *box,
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT UnlockBox(
|
|
);
|
|
HRESULT LoadTexture(
|
|
[in] int gl_level,
|
|
[in] BOOL srgb_mode
|
|
);
|
|
HRESULT SetContainer(
|
|
[in] IWineD3DBase *container
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(3c2aebf6-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DBaseTexture : IWineD3DResource
|
|
{
|
|
DWORD SetLOD(
|
|
[in] DWORD new_lod
|
|
);
|
|
DWORD GetLOD(
|
|
);
|
|
DWORD GetLevelCount(
|
|
);
|
|
HRESULT SetAutoGenFilterType(
|
|
WINED3DTEXTUREFILTERTYPE filter_type
|
|
);
|
|
WINED3DTEXTUREFILTERTYPE GetAutoGenFilterType(
|
|
);
|
|
void GenerateMipSubLevels(
|
|
);
|
|
BOOL SetDirty(
|
|
BOOL dirty
|
|
);
|
|
BOOL GetDirty(
|
|
);
|
|
HRESULT BindTexture(
|
|
);
|
|
UINT GetTextureDimensions(
|
|
);
|
|
BOOL IsCondNP2(
|
|
);
|
|
void ApplyStateChanges(
|
|
const DWORD texture_states[WINED3D_HIGHEST_TEXTURE_STATE + 1],
|
|
const DWORD sampler_states[WINED3D_HIGHEST_SAMPLER_STATE + 1]
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(3e72cc1c-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DTexture : IWineD3DBaseTexture
|
|
{
|
|
void Destroy(
|
|
[in] D3DCB_DESTROYSURFACEFN destroy_surface_callback
|
|
);
|
|
HRESULT GetLevelDesc(
|
|
[in] UINT level,
|
|
[out] WINED3DSURFACE_DESC *desc
|
|
);
|
|
HRESULT GetSurfaceLevel(
|
|
[in] UINT level,
|
|
[out] IWineD3DSurface **surface
|
|
);
|
|
HRESULT LockRect(
|
|
[in] UINT level,
|
|
[out] WINED3DLOCKED_RECT *locked_rect,
|
|
[in] const RECT *rect,
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT UnlockRect(
|
|
[in] UINT level
|
|
);
|
|
HRESULT AddDirtyRect(
|
|
[in] const RECT *dirty_rect
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(41752900-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DCubeTexture : IWineD3DBaseTexture
|
|
{
|
|
void Destroy(
|
|
[in] D3DCB_DESTROYSURFACEFN destroy_surface_callback
|
|
);
|
|
HRESULT GetLevelDesc(
|
|
[in] UINT level,
|
|
[out] WINED3DSURFACE_DESC *desc
|
|
);
|
|
HRESULT GetCubeMapSurface(
|
|
[in] WINED3DCUBEMAP_FACES face,
|
|
[in] UINT level,
|
|
[out] IWineD3DSurface **surface
|
|
);
|
|
HRESULT LockRect(
|
|
[in] WINED3DCUBEMAP_FACES face,
|
|
[in] UINT level,
|
|
[out] WINED3DLOCKED_RECT *locked_rect,
|
|
[in] const RECT *rect,
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT UnlockRect(
|
|
[in] WINED3DCUBEMAP_FACES face,
|
|
[in] UINT level
|
|
);
|
|
HRESULT AddDirtyRect(
|
|
[in] WINED3DCUBEMAP_FACES face,
|
|
[in] const RECT *dirty_rect
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(7b39470c-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DVolumeTexture : IWineD3DBaseTexture
|
|
{
|
|
void Destroy(
|
|
[in] D3DCB_DESTROYVOLUMEFN destroy_volume_callback
|
|
);
|
|
HRESULT GetLevelDesc(
|
|
[in] UINT level,
|
|
[out] WINED3DVOLUME_DESC *desc
|
|
);
|
|
HRESULT GetVolumeLevel(
|
|
[in] UINT level,
|
|
[out] IWineD3DVolume **volume
|
|
);
|
|
HRESULT LockBox(
|
|
[in] UINT level,
|
|
[out] WINED3DLOCKED_BOX *locked_box,
|
|
[in] const WINED3DBOX *box,
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT UnlockBox(
|
|
[in] UINT level
|
|
);
|
|
HRESULT AddDirtyBox(
|
|
[in] const WINED3DBOX *dirty_box
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(7cd55be6-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DVertexDeclaration : IWineD3DBase
|
|
{
|
|
HRESULT GetDevice(
|
|
[out] IWineD3DDevice **device
|
|
);
|
|
HRESULT GetDeclaration(
|
|
[out] WINED3DVERTEXELEMENT *elements,
|
|
[out] UINT *element_count
|
|
);
|
|
HRESULT SetDeclaration(
|
|
[in] const WINED3DVERTEXELEMENT *elements,
|
|
[in] UINT element_count
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(83b073ce-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DStateBlock : IWineD3DBase
|
|
{
|
|
HRESULT GetDevice(
|
|
[out] IWineD3DDevice **device
|
|
);
|
|
HRESULT Capture(
|
|
);
|
|
HRESULT Apply(
|
|
);
|
|
HRESULT InitStartupStateBlock(
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(905ddbac-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DQuery : IWineD3DBase
|
|
{
|
|
HRESULT GetDevice(
|
|
[out] IWineD3DDevice **device
|
|
);
|
|
HRESULT GetData(
|
|
[out] void *data,
|
|
[in] DWORD data_size,
|
|
[in] DWORD flags
|
|
);
|
|
DWORD GetDataSize(
|
|
);
|
|
WINED3DQUERYTYPE GetType(
|
|
);
|
|
HRESULT Issue(
|
|
DWORD flags
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(34d01b10-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DSwapChain : IWineD3DBase
|
|
{
|
|
void Destroy(
|
|
[in] D3DCB_DESTROYSURFACEFN destroy_surface_callback
|
|
);
|
|
HRESULT GetDevice(
|
|
[out] IWineD3DDevice **device
|
|
);
|
|
HRESULT Present(
|
|
[in] const RECT *src_rect,
|
|
[in] const RECT *dst_rect,
|
|
[in] HWND dst_window_override,
|
|
[in] const RGNDATA *dirty_region,
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT SetDestWindowOverride(
|
|
[in] HWND window
|
|
);
|
|
HRESULT GetFrontBufferData(
|
|
[in] IWineD3DSurface *dst_surface
|
|
);
|
|
HRESULT GetBackBuffer(
|
|
[in] UINT backbuffer_idx,
|
|
[in] WINED3DBACKBUFFER_TYPE backbuffer_type,
|
|
[out] IWineD3DSurface **backbuffer
|
|
);
|
|
HRESULT GetRasterStatus(
|
|
[out] WINED3DRASTER_STATUS *raster_status
|
|
);
|
|
HRESULT GetDisplayMode(
|
|
[out] WINED3DDISPLAYMODE *mode
|
|
);
|
|
HRESULT GetPresentParameters(
|
|
[out] WINED3DPRESENT_PARAMETERS *present_parameters
|
|
);
|
|
HRESULT SetGammaRamp(
|
|
[in] DWORD flags,
|
|
[in] const WINED3DGAMMARAMP *ramp
|
|
);
|
|
HRESULT GetGammaRamp(
|
|
[out] WINED3DGAMMARAMP *ramp
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(eac93065-a4df-446f-86a1-9ef2bca40a3c)
|
|
]
|
|
interface IWineD3DBaseShader : IWineD3DBase
|
|
{
|
|
HRESULT SetFunction(
|
|
[in] const DWORD *function
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(7f7a2b60-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DVertexShader : IWineD3DBaseShader
|
|
{
|
|
HRESULT GetDevice(
|
|
[out] IWineD3DDevice **device
|
|
);
|
|
HRESULT GetFunction(
|
|
[out] void *data,
|
|
[in, out] UINT *data_size
|
|
);
|
|
void FakeSemantics(
|
|
[in] IWineD3DVertexDeclaration *vertex_declaration
|
|
);
|
|
HRESULT SetLocalConstantsF(
|
|
[in] UINT start_idx,
|
|
[in] const float *src_data,
|
|
[in] UINT vector4f_count
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(818503da-6f30-11d9-c687-00046142c14f)
|
|
]
|
|
interface IWineD3DPixelShader : IWineD3DBaseShader
|
|
{
|
|
HRESULT GetDevice(
|
|
[out] IWineD3DDevice **device
|
|
);
|
|
HRESULT GetFunction(
|
|
[out] void *data,
|
|
[in, out] UINT *data_size
|
|
);
|
|
}
|
|
|
|
[
|
|
object,
|
|
local,
|
|
uuid(6d10a2ce-09d0-4a53-a427-11388f9f8ca5)
|
|
]
|
|
interface IWineD3DDevice : IWineD3DBase
|
|
{
|
|
HRESULT CreateVertexBuffer(
|
|
[in] UINT length,
|
|
[in] DWORD usage,
|
|
[in] DWORD fvf,
|
|
[in] WINED3DPOOL pool,
|
|
[out] IWineD3DVertexBuffer **vertex_buffer,
|
|
[in] HANDLE *shared_handle,
|
|
[in] IUnknown *parent
|
|
);
|
|
HRESULT CreateIndexBuffer(
|
|
[in] UINT length,
|
|
[in] DWORD usage,
|
|
[in] WINED3DFORMAT format,
|
|
[in] WINED3DPOOL pool,
|
|
[out] IWineD3DIndexBuffer **index_buffer,
|
|
[in] HANDLE *shared_handle,
|
|
[in] IUnknown *parent
|
|
);
|
|
HRESULT CreateStateBlock(
|
|
[in] WINED3DSTATEBLOCKTYPE type,
|
|
[out] IWineD3DStateBlock **stateblock,
|
|
[in] IUnknown *parent
|
|
);
|
|
HRESULT CreateSurface(
|
|
[in] UINT width,
|
|
[in] UINT height,
|
|
[in] WINED3DFORMAT format,
|
|
[in] BOOL lockable,
|
|
[in] BOOL discard,
|
|
[in] UINT level,
|
|
[out] IWineD3DSurface **surface,
|
|
[in] WINED3DRESOURCETYPE resource_type,
|
|
[in] DWORD usage,
|
|
[in] WINED3DPOOL pool,
|
|
[in] WINED3DMULTISAMPLE_TYPE multisample_type,
|
|
[in] DWORD multisample_quality,
|
|
[in] HANDLE *shared_handle,
|
|
[in] WINED3DSURFTYPE surface_type,
|
|
[in] IUnknown *parent
|
|
);
|
|
HRESULT CreateTexture(
|
|
[in] UINT width,
|
|
[in] UINT height,
|
|
[in] UINT levels,
|
|
[in] DWORD usage,
|
|
[in] WINED3DFORMAT format,
|
|
[in] WINED3DPOOL pool,
|
|
[out] IWineD3DTexture **texture,
|
|
[in] HANDLE *shared_handle,
|
|
[in] IUnknown *parent
|
|
);
|
|
HRESULT CreateVolumeTexture(
|
|
[in] UINT width,
|
|
[in] UINT height,
|
|
[in] UINT depth,
|
|
[in] UINT levels,
|
|
[in] DWORD usage,
|
|
[in] WINED3DFORMAT format,
|
|
[in] WINED3DPOOL pool,
|
|
[out] IWineD3DVolumeTexture **texture,
|
|
[in] HANDLE *shared_handle,
|
|
[in] IUnknown *parent
|
|
);
|
|
HRESULT CreateVolume(
|
|
[in] UINT width,
|
|
[in] UINT height,
|
|
[in] UINT depth,
|
|
[in] DWORD usage,
|
|
[in] WINED3DFORMAT format,
|
|
[in] WINED3DPOOL pool,
|
|
[out] IWineD3DVolume **volume,
|
|
[in] HANDLE *shared_handle,
|
|
[in] IUnknown *parent
|
|
);
|
|
HRESULT CreateCubeTexture(
|
|
[in] UINT edge_length,
|
|
[in] UINT levels,
|
|
[in] DWORD usage,
|
|
[in] WINED3DFORMAT format,
|
|
[in] WINED3DPOOL pool,
|
|
[out] IWineD3DCubeTexture **texture,
|
|
[in] HANDLE *shared_handle,
|
|
[in] IUnknown *parent
|
|
);
|
|
HRESULT CreateQuery(
|
|
[in] WINED3DQUERYTYPE type,
|
|
[out] IWineD3DQuery **query,
|
|
[in] IUnknown *parent
|
|
);
|
|
HRESULT CreateSwapChain(
|
|
[in] WINED3DPRESENT_PARAMETERS *present_parameters,
|
|
[out] IWineD3DSwapChain **swapchain,
|
|
[in] IUnknown *parent,
|
|
[in] WINED3DSURFTYPE surface_type
|
|
);
|
|
HRESULT CreateVertexDeclaration(
|
|
[out] IWineD3DVertexDeclaration **declaration,
|
|
[in] IUnknown *parent,
|
|
[in] const WINED3DVERTEXELEMENT *elements,
|
|
[in] UINT element_count
|
|
);
|
|
HRESULT CreateVertexDeclarationFromFVF(
|
|
[out] IWineD3DVertexDeclaration **declaration,
|
|
[in] IUnknown *parent,
|
|
[in] DWORD fvf
|
|
);
|
|
HRESULT CreateVertexShader(
|
|
[in] IWineD3DVertexDeclaration *declaration,
|
|
[in] const DWORD *function,
|
|
[out] IWineD3DVertexShader **shader,
|
|
[in] IUnknown *parent
|
|
);
|
|
HRESULT CreatePixelShader(
|
|
[in] const DWORD *function,
|
|
[out] IWineD3DPixelShader **shader,
|
|
[in] IUnknown *parent
|
|
);
|
|
HRESULT CreatePalette(
|
|
[in] DWORD flags,
|
|
[in] const PALETTEENTRY *palette_entry,
|
|
[out] IWineD3DPalette **palette,
|
|
[in] IUnknown *parent
|
|
);
|
|
HRESULT Init3D(
|
|
[in] WINED3DPRESENT_PARAMETERS *present_parameters
|
|
);
|
|
HRESULT InitGDI(
|
|
[in] WINED3DPRESENT_PARAMETERS *present_parameters
|
|
);
|
|
HRESULT Uninit3D(
|
|
[in] D3DCB_DESTROYSURFACEFN destroy_surface_callback,
|
|
[in] D3DCB_DESTROYSWAPCHAINFN destroy_swapchain_callback
|
|
);
|
|
HRESULT UninitGDI(
|
|
[in] D3DCB_DESTROYSWAPCHAINFN destroy_swapchain_callback
|
|
);
|
|
void SetMultithreaded(
|
|
);
|
|
HRESULT EvictManagedResources(
|
|
);
|
|
UINT GetAvailableTextureMem(
|
|
);
|
|
HRESULT GetBackBuffer(
|
|
[in] UINT swapchain_idx,
|
|
[in] UINT backbuffer_idx,
|
|
[in] WINED3DBACKBUFFER_TYPE backbuffer_type,
|
|
[out] IWineD3DSurface **backbuffer
|
|
);
|
|
HRESULT GetCreationParameters(
|
|
[out] WINED3DDEVICE_CREATION_PARAMETERS *creation_parameters
|
|
);
|
|
HRESULT GetDeviceCaps(
|
|
[out] WINED3DCAPS *caps
|
|
);
|
|
HRESULT GetDirect3D(
|
|
[out] IWineD3D** d3d
|
|
);
|
|
HRESULT GetDisplayMode(
|
|
[in] UINT swapchain_idx,
|
|
[out] WINED3DDISPLAYMODE *mode
|
|
);
|
|
HRESULT SetDisplayMode(
|
|
[in] UINT swapchain_idx,
|
|
[in] const WINED3DDISPLAYMODE *mode
|
|
);
|
|
UINT GetNumberOfSwapChains(
|
|
);
|
|
HRESULT GetRasterStatus(
|
|
[in] UINT swapchain_idx,
|
|
[out] WINED3DRASTER_STATUS *raster_status
|
|
);
|
|
HRESULT GetSwapChain(
|
|
[in] UINT swapchain_idx,
|
|
[out] IWineD3DSwapChain **swapchain
|
|
);
|
|
HRESULT Reset(
|
|
[in] WINED3DPRESENT_PARAMETERS *present_parameters
|
|
);
|
|
HRESULT SetDialogBoxMode(
|
|
[in] BOOL enable_dialogs
|
|
);
|
|
HRESULT SetCursorProperties(
|
|
[in] UINT x_hotspot,
|
|
[in] UINT y_hotspot,
|
|
[in] IWineD3DSurface *cursor_surface
|
|
);
|
|
void SetCursorPosition(
|
|
[in] int x_screen_space,
|
|
[in] int y_screen_space,
|
|
[in] DWORD flags
|
|
);
|
|
BOOL ShowCursor(
|
|
[in] BOOL show
|
|
);
|
|
HRESULT TestCooperativeLevel(
|
|
);
|
|
HRESULT SetClipPlane(
|
|
[in] DWORD plane_idx,
|
|
[in] const float *plane
|
|
);
|
|
HRESULT GetClipPlane(
|
|
[in] DWORD plane_idx,
|
|
[out] float *plane
|
|
);
|
|
HRESULT SetClipStatus(
|
|
[in] const WINED3DCLIPSTATUS *clip_status
|
|
);
|
|
HRESULT GetClipStatus(
|
|
[out] WINED3DCLIPSTATUS *clip_status
|
|
);
|
|
HRESULT SetCurrentTexturePalette(
|
|
[in] UINT palette_number
|
|
);
|
|
HRESULT GetCurrentTexturePalette(
|
|
[out] UINT *palette_number
|
|
);
|
|
HRESULT SetDepthStencilSurface(
|
|
[in] IWineD3DSurface *depth_stencil
|
|
);
|
|
HRESULT GetDepthStencilSurface(
|
|
[out] IWineD3DSurface **depth_stencil
|
|
);
|
|
void SetGammaRamp(
|
|
[in] UINT swapchain_idx,
|
|
[in] DWORD flags,
|
|
[in] const WINED3DGAMMARAMP *ramp
|
|
);
|
|
void GetGammaRamp(
|
|
[in] UINT swapchain_idx,
|
|
[out] WINED3DGAMMARAMP *ramp
|
|
);
|
|
HRESULT SetIndices(
|
|
[in] IWineD3DIndexBuffer *index_buffer
|
|
);
|
|
HRESULT GetIndices(
|
|
[out] IWineD3DIndexBuffer **index_buffer
|
|
);
|
|
HRESULT SetBaseVertexIndex(
|
|
[in] INT base_index
|
|
);
|
|
HRESULT GetBaseVertexIndex(
|
|
[out] INT *base_index
|
|
);
|
|
HRESULT SetLight(
|
|
[in] DWORD light_idx,
|
|
[in] const WINED3DLIGHT *light
|
|
);
|
|
HRESULT GetLight(
|
|
[in] DWORD light_idx,
|
|
[out] WINED3DLIGHT *light
|
|
);
|
|
HRESULT SetLightEnable(
|
|
[in] DWORD light_idx,
|
|
[in] BOOL enable
|
|
);
|
|
HRESULT GetLightEnable(
|
|
[in] DWORD light_idx,
|
|
[out] BOOL *enable
|
|
);
|
|
HRESULT SetMaterial(
|
|
[in] const WINED3DMATERIAL *material
|
|
);
|
|
HRESULT GetMaterial(
|
|
[out] WINED3DMATERIAL *material
|
|
);
|
|
HRESULT SetNPatchMode(
|
|
[in] float segments
|
|
);
|
|
float GetNPatchMode(
|
|
);
|
|
HRESULT SetPaletteEntries(
|
|
[in] UINT palette_number,
|
|
[in] const PALETTEENTRY *entries
|
|
);
|
|
HRESULT GetPaletteEntries(
|
|
[in] UINT palette_number,
|
|
[out] PALETTEENTRY *entries
|
|
);
|
|
HRESULT SetPixelShader(
|
|
[in] IWineD3DPixelShader *shader
|
|
);
|
|
HRESULT GetPixelShader(
|
|
[out] IWineD3DPixelShader **shader
|
|
);
|
|
HRESULT SetPixelShaderConstantB(
|
|
[in] UINT start_register,
|
|
[in] const BOOL *constants,
|
|
[in] UINT bool_count
|
|
);
|
|
HRESULT GetPixelShaderConstantB(
|
|
[in] UINT start_register,
|
|
[out] BOOL *constants,
|
|
[in] UINT bool_count
|
|
);
|
|
HRESULT SetPixelShaderConstantI(
|
|
[in] UINT start_register,
|
|
[in] const int *constants,
|
|
[in] UINT vector4i_count
|
|
);
|
|
HRESULT GetPixelShaderConstantI(
|
|
[in] UINT start_register,
|
|
[out] int *constants,
|
|
[in] UINT vector4i_count
|
|
);
|
|
HRESULT SetPixelShaderConstantF(
|
|
[in] UINT start_register,
|
|
[in] const float *constants,
|
|
[in] UINT vector4f_count
|
|
);
|
|
HRESULT GetPixelShaderConstantF(
|
|
[in] UINT start_register,
|
|
[out] float *constants,
|
|
[in] UINT vector4f_count
|
|
);
|
|
HRESULT SetRenderState(
|
|
[in] WINED3DRENDERSTATETYPE state,
|
|
[in] DWORD value
|
|
);
|
|
HRESULT GetRenderState(
|
|
[in] WINED3DRENDERSTATETYPE state,
|
|
[out] DWORD *value
|
|
);
|
|
HRESULT SetRenderTarget(
|
|
[in] DWORD render_target_idx,
|
|
[in] IWineD3DSurface *render_target
|
|
);
|
|
HRESULT GetRenderTarget(
|
|
[in] DWORD render_target_idx,
|
|
[out] IWineD3DSurface **render_target
|
|
);
|
|
HRESULT SetFrontBackBuffers(
|
|
[in] IWineD3DSurface *front,
|
|
[in] IWineD3DSurface *back
|
|
);
|
|
HRESULT SetSamplerState(
|
|
[in] DWORD sampler_idx,
|
|
[in] WINED3DSAMPLERSTATETYPE state,
|
|
[in] DWORD value
|
|
);
|
|
HRESULT GetSamplerState(
|
|
[in] DWORD sampler_idx,
|
|
[in] WINED3DSAMPLERSTATETYPE state,
|
|
[out] DWORD *value
|
|
);
|
|
HRESULT SetScissorRect(
|
|
[in] const RECT *rect
|
|
);
|
|
HRESULT GetScissorRect(
|
|
[out] RECT *rect
|
|
);
|
|
HRESULT SetSoftwareVertexProcessing(
|
|
[in] BOOL software
|
|
);
|
|
BOOL GetSoftwareVertexProcessing(
|
|
);
|
|
HRESULT SetStreamSource(
|
|
[in] UINT stream_idx,
|
|
[in] IWineD3DVertexBuffer *vertex_buffer,
|
|
[in] UINT offset,
|
|
[in] UINT stride
|
|
);
|
|
HRESULT GetStreamSource(
|
|
[in] UINT stream_idx,
|
|
[out] IWineD3DVertexBuffer **vertex_buffer,
|
|
[out] UINT *offset,
|
|
[out] UINT *stride
|
|
);
|
|
HRESULT SetStreamSourceFreq(
|
|
[in] UINT stream_idx,
|
|
[in] UINT divider
|
|
);
|
|
HRESULT GetStreamSourceFreq(
|
|
[in] UINT stream_idx,
|
|
[out] UINT *divider
|
|
);
|
|
HRESULT SetTexture(
|
|
[in] DWORD stage,
|
|
[in] IWineD3DBaseTexture *texture
|
|
);
|
|
HRESULT GetTexture(
|
|
[in] DWORD stage,
|
|
[out] IWineD3DBaseTexture **texture
|
|
);
|
|
HRESULT SetTextureStageState(
|
|
[in] DWORD stage,
|
|
[in] WINED3DTEXTURESTAGESTATETYPE state,
|
|
[in] DWORD value
|
|
);
|
|
HRESULT GetTextureStageState(
|
|
[in] DWORD stage,
|
|
[in] WINED3DTEXTURESTAGESTATETYPE state,
|
|
[out] DWORD *value
|
|
);
|
|
HRESULT SetTransform(
|
|
[in] WINED3DTRANSFORMSTATETYPE state,
|
|
[in] const WINED3DMATRIX *matrix
|
|
);
|
|
HRESULT GetTransform(
|
|
[in] WINED3DTRANSFORMSTATETYPE state,
|
|
[out] WINED3DMATRIX *matrix
|
|
);
|
|
HRESULT SetVertexDeclaration(
|
|
[in] IWineD3DVertexDeclaration *declaration
|
|
);
|
|
HRESULT GetVertexDeclaration(
|
|
[out] IWineD3DVertexDeclaration **declaration
|
|
);
|
|
HRESULT SetVertexShader(
|
|
[in] IWineD3DVertexShader *shader
|
|
);
|
|
HRESULT GetVertexShader(
|
|
[out] IWineD3DVertexShader **shader
|
|
);
|
|
HRESULT SetVertexShaderConstantB(
|
|
[in] UINT start_register,
|
|
[in] const BOOL *constants,
|
|
[in] UINT bool_count
|
|
);
|
|
HRESULT GetVertexShaderConstantB(
|
|
[in] UINT start_register,
|
|
[out] BOOL *constants,
|
|
[in] UINT bool_count
|
|
);
|
|
HRESULT SetVertexShaderConstantI(
|
|
[in] UINT start_register,
|
|
[in] const int *constants,
|
|
[in] UINT vector4i_count
|
|
);
|
|
HRESULT GetVertexShaderConstantI(
|
|
[in] UINT start_register,
|
|
[out] int *constants,
|
|
[in] UINT vector4i_count
|
|
);
|
|
HRESULT SetVertexShaderConstantF(
|
|
[in] UINT start_register,
|
|
[in] const float *constants,
|
|
[in] UINT vector4f_count
|
|
);
|
|
HRESULT GetVertexShaderConstantF(
|
|
[in] UINT start_register,
|
|
[out] float *constants,
|
|
[in] UINT vector4f_count
|
|
);
|
|
HRESULT SetViewport(
|
|
[in] const WINED3DVIEWPORT *viewport
|
|
);
|
|
HRESULT GetViewport(
|
|
[out] WINED3DVIEWPORT *viewport
|
|
);
|
|
HRESULT MultiplyTransform(
|
|
[in] WINED3DTRANSFORMSTATETYPE state,
|
|
[in] const WINED3DMATRIX *matrix
|
|
);
|
|
HRESULT ValidateDevice(
|
|
[out] DWORD *num_passes
|
|
);
|
|
HRESULT ProcessVertices(
|
|
[in] UINT src_start_idx,
|
|
[in] UINT dst_idx,
|
|
[in] UINT vertex_count,
|
|
[in] IWineD3DVertexBuffer *dest_buffer,
|
|
[in] IWineD3DVertexDeclaration *declaration,
|
|
[in] DWORD flags
|
|
);
|
|
HRESULT BeginStateBlock(
|
|
);
|
|
HRESULT EndStateBlock(
|
|
[out] IWineD3DStateBlock **stateblock
|
|
);
|
|
HRESULT BeginScene(
|
|
);
|
|
HRESULT EndScene(
|
|
);
|
|
HRESULT Present(
|
|
[in] const RECT *src_rect,
|
|
[in] const RECT *dst_rect,
|
|
[in] HWND dst_window_override,
|
|
[in] const RGNDATA *dirty_region
|
|
);
|
|
HRESULT Clear(
|
|
[in] DWORD rect_count,
|
|
[in] const WINED3DRECT *rects,
|
|
[in] DWORD flags,
|
|
[in] WINED3DCOLOR color,
|
|
[in] float z,
|
|
[in] DWORD stencil
|
|
);
|
|
HRESULT DrawPrimitive(
|
|
[in] WINED3DPRIMITIVETYPE primitive_type,
|
|
[in] UINT start_vertex,
|
|
[in] UINT primitive_count
|
|
);
|
|
HRESULT DrawIndexedPrimitive(
|
|
[in] WINED3DPRIMITIVETYPE primitive_type,
|
|
[in] UINT min_vertex_idx,
|
|
[in] UINT vertex_count,
|
|
[in] UINT start_idx,
|
|
[in] UINT primitive_count
|
|
);
|
|
HRESULT DrawPrimitiveUP(
|
|
[in] WINED3DPRIMITIVETYPE primitive_type,
|
|
[in] UINT primitive_count,
|
|
[in] const void *stream_data,
|
|
[in] UINT stream_stride
|
|
);
|
|
HRESULT DrawIndexedPrimitiveUP(
|
|
[in] WINED3DPRIMITIVETYPE primitive_type,
|
|
[in] UINT min_vertex_idx,
|
|
[in] UINT vertex_count,
|
|
[in] UINT primitive_count,
|
|
[in] const void *index_data,
|
|
[in] WINED3DFORMAT index_data_format,
|
|
[in] const void *stream_data,
|
|
[in] UINT stream_stride
|
|
);
|
|
HRESULT DrawPrimitiveStrided(
|
|
[in] WINED3DPRIMITIVETYPE primitive_type,
|
|
[in] UINT primitive_count,
|
|
[in] const WineDirect3DVertexStridedData *strided_data
|
|
);
|
|
HRESULT DrawIndexedPrimitiveStrided(
|
|
[in] WINED3DPRIMITIVETYPE primitive_type,
|
|
[in] UINT primitive_count,
|
|
[in] const WineDirect3DVertexStridedData *strided_data,
|
|
[in] UINT vertex_count,
|
|
[in] const void *index_data,
|
|
[in] WINED3DFORMAT index_data_format
|
|
);
|
|
HRESULT DrawRectPatch(
|
|
[in] UINT handle,
|
|
[in] const float *num_segs,
|
|
[in] const WINED3DRECTPATCH_INFO *rect_patch_info
|
|
);
|
|
HRESULT DrawTriPatch(
|
|
[in] UINT handle,
|
|
[in] const float *num_segs,
|
|
[in] const WINED3DTRIPATCH_INFO *tri_patch_info
|
|
);
|
|
HRESULT DeletePatch(
|
|
[in] UINT handle
|
|
);
|
|
HRESULT ColorFill(
|
|
[in] IWineD3DSurface *surface,
|
|
[in] const WINED3DRECT *rect,
|
|
[in] WINED3DCOLOR color
|
|
);
|
|
HRESULT UpdateTexture(
|
|
[in] IWineD3DBaseTexture *src_texture,
|
|
[in] IWineD3DBaseTexture *dst_texture
|
|
);
|
|
HRESULT UpdateSurface(
|
|
[in] IWineD3DSurface *src_surface,
|
|
[in] const RECT *src_rect,
|
|
[in] IWineD3DSurface *dst_surface,
|
|
[in] const POINT *dst_point
|
|
);
|
|
HRESULT GetFrontBufferData(
|
|
[in] UINT swapchain_idx,
|
|
[in] IWineD3DSurface *dst_surface
|
|
);
|
|
void ResourceReleased(
|
|
[in] IWineD3DResource *resource
|
|
);
|
|
HRESULT EnumResources(
|
|
[in] D3DCB_ENUMRESOURCES callback,
|
|
[in] void *data
|
|
);
|
|
}
|
|
|
|
IWineD3D *WineDirect3DCreate(UINT dxVersion, IUnknown *parent);
|
|
IWineD3DClipper *WineDirect3DCreateClipper(IUnknown *parent);
|