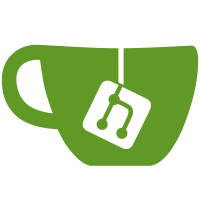
Sun Aug 11 13:00:20 1996 Alexandre Julliard <julliard@lrc.epfl.ch> * [configure.in] [include/acconfig.h] [tools/build.c] Added check for underscore on external symbols. * [memory/selector.c] [memory/global.c] Fixed FreeSelector() to free only one selector. Added SELECTOR_FreeBlock() to free an array of selectors. * [objects/color.c] Fixed a bug in COLOR_ToLogical() that caused GetPixel() to fail on hi-color displays. * [tools/build.c] [if1632/crtdll.spec] Added 'extern' type, used for external variables or functions. * [windows/winpos.c] Allow de-activating a window in WINPOS_ChangeActiveWindow(). * [windows/winproc.c] Added 32-to-16 translation for button messages. Fixed WINPROC_GetPtr() to avoid crashes on 32-bit procedures that happen to be valid SEGPTRs. Sat Aug 10 18:22:25 1996 Albrecht Kleine <kleine@ak.sax.de> * [windows/message.c] Removed a FIXME in MSG_PeekHardwareMsg(): produces correct data for the JOURNALRECORD-hook (using EVENTMSG16 structure). * [if1632/gdi.spec] [include/windows.h] [objects/metafile.c] Introduced undocumented API function IsValidMetaFile(), plus a minor fix in last patch of CopyMetaFile(). * [objects/gdiobj.c] Removed a FIXME in IsGDIObject(): added magic word check. Sun Aug 10 18:10:10 1996 Bruce Milner <Bruce.Milner@genetics.utah.edu> * [controls/statuswin.c] First pass at implementing the StatusWindow class. * [include/commctrl.h] Header file for common controls. * [controls/widgets.c] Added InitCommonControls(). * [if1632/comctl32.spec] Add DrawStatusTextA, CreateStatusWindowA, InitCommonControls. * [win32/findfile.c] [if1632/kernel32.spec] Add FindNextFile32A, FindClose. Modified FindFirstFile32A so it works with FindNextFile32A. * [include/winbase.h] Fixed WIN32_FIND_DATA structure member names. Sat Aug 10 09:00:00 1996 Alex Korobka <alex@phm30.pharm.sunysb.edu> * [windows/scroll.c] Changed scrolling routines to benefit from DCE code update. Thu Aug 8 18:05:09 1996 Marcus Meissner <msmeissn@cip.informatik.uni-erlangen.de> * [files/file.c] SearchPath* could get NULL for lastpart argument. * [if1632/build-spec.txt] [documentation/debugging] Varargs documentation added, debugging hints updated. * [if1632/crtdll.spec][misc/crtdll.c][misc/Makefile.in] Started to implement CRTDLL. * [if1632/wsock32.spec] Some thunks to standard libc functions (structures have the same elements, but perhaps wrong offset due to packing). * [include/kernel32.h][include/windows.h][win32/*.c][loader/main.c] Merged kernel32.h into windows.h. * [misc/lstr.c] Enhanced FormatMessage(). * [misc/main.c] [if1632/kernel.spec] [include/windows.h] GetVersion() updated to new naming standard. Changed language handling to support language ids. * [misc/shell.c] Enhanced FindExecutable, so it finds files in the search path too. * [win32/environment.c] GetCommandLine* updated. * [loader/resource.c] [loader/pe_resource.c] FindResourceEx32* added. Loading of messagetables added. Language handling now uses Wine default language id.
144 lines
4.3 KiB
C
144 lines
4.3 KiB
C
/*
|
|
* Windows widgets (built-in window classes)
|
|
*
|
|
* Copyright 1993 Alexandre Julliard
|
|
*/
|
|
|
|
#include "win.h"
|
|
#include "commctrl.h"
|
|
#include "button.h"
|
|
#include "static.h"
|
|
#include "status.h"
|
|
#include "scroll.h"
|
|
#include "desktop.h"
|
|
#include "mdi.h"
|
|
#include "gdi.h"
|
|
#include "user.h"
|
|
#include "module.h"
|
|
#include "heap.h"
|
|
|
|
typedef struct
|
|
{
|
|
UINT16 style;
|
|
INT16 wndExtra;
|
|
HBRUSH16 background;
|
|
LPCSTR procName;
|
|
LPCSTR className;
|
|
} BUILTIN_CLASS_INFO16;
|
|
|
|
static const BUILTIN_CLASS_INFO16 WIDGETS_BuiltinClasses16[] =
|
|
{
|
|
{ CS_GLOBALCLASS | CS_PARENTDC,
|
|
sizeof(STATICINFO), 0, "StaticWndProc", "STATIC" },
|
|
{ CS_GLOBALCLASS | CS_DBLCLKS | CS_VREDRAW | CS_HREDRAW | CS_PARENTDC,
|
|
sizeof(SCROLLINFO), 0, "ScrollBarWndProc", "SCROLLBAR" },
|
|
{ CS_GLOBALCLASS | CS_PARENTDC | CS_DBLCLKS,
|
|
8, 0, "ListBoxWndProc", "LISTBOX" },
|
|
{ CS_GLOBALCLASS | CS_PARENTDC | CS_DBLCLKS,
|
|
8, 0, "ComboBoxWndProc", "COMBOBOX" },
|
|
{ CS_GLOBALCLASS | CS_DBLCLKS | CS_SAVEBITS,
|
|
8, 0, "ComboLBoxWndProc", "COMBOLBOX" },
|
|
{ CS_GLOBALCLASS | CS_PARENTDC | CS_DBLCLKS,
|
|
sizeof(DWORD), 0, "EditWndProc", "EDIT" },
|
|
{ CS_GLOBALCLASS | CS_SAVEBITS,
|
|
sizeof(HMENU32), 0, "PopupMenuWndProc", POPUPMENU_CLASS_NAME },
|
|
{ CS_GLOBALCLASS | CS_SAVEBITS,
|
|
DLGWINDOWEXTRA, 0, "DefDlgProc", DIALOG_CLASS_NAME },
|
|
{ CS_GLOBALCLASS, sizeof(MDICLIENTINFO),
|
|
STOCK_LTGRAY_BRUSH, "MDIClientWndProc", "MDICLIENT" }
|
|
};
|
|
|
|
#define NB_BUILTIN_CLASSES16 \
|
|
(sizeof(WIDGETS_BuiltinClasses16)/sizeof(WIDGETS_BuiltinClasses16[0]))
|
|
|
|
|
|
static WNDCLASS32A WIDGETS_BuiltinClasses32[] =
|
|
{
|
|
{ CS_GLOBALCLASS | CS_DBLCLKS | CS_VREDRAW | CS_HREDRAW | CS_PARENTDC,
|
|
ButtonWndProc, 0, sizeof(BUTTONINFO), 0, 0, 0, 0, 0, "BUTTON" },
|
|
{ CS_GLOBALCLASS, DesktopWndProc, 0, sizeof(DESKTOPINFO),
|
|
0, 0, 0, 0, 0, DESKTOP_CLASS_NAME }
|
|
};
|
|
|
|
#define NB_BUILTIN_CLASSES32 \
|
|
(sizeof(WIDGETS_BuiltinClasses32)/sizeof(WIDGETS_BuiltinClasses32[0]))
|
|
|
|
|
|
static WNDCLASS32A WIDGETS_CommonControls32[] =
|
|
{
|
|
{ CS_GLOBALCLASS | CS_VREDRAW | CS_HREDRAW, StatusWindowProc, 0,
|
|
sizeof(STATUSWINDOWINFO), 0, 0, 0, 0, 0, STATUSCLASSNAME32A },
|
|
};
|
|
|
|
#define NB_COMMON_CONTROLS32 \
|
|
(sizeof(WIDGETS_CommonControls32)/sizeof(WIDGETS_CommonControls32[0]))
|
|
|
|
|
|
/***********************************************************************
|
|
* WIDGETS_Init
|
|
*
|
|
* Initialize the built-in window classes.
|
|
*/
|
|
BOOL WIDGETS_Init(void)
|
|
{
|
|
int i;
|
|
char *name;
|
|
const BUILTIN_CLASS_INFO16 *info16 = WIDGETS_BuiltinClasses16;
|
|
WNDCLASS16 class16;
|
|
WNDCLASS32A *class32 = WIDGETS_BuiltinClasses32;
|
|
|
|
if (!(name = SEGPTR_ALLOC( 20 * sizeof(char) ))) return FALSE;
|
|
|
|
/* Create 16-bit classes */
|
|
|
|
class16.cbClsExtra = 0;
|
|
class16.hInstance = 0;
|
|
class16.hIcon = 0;
|
|
class16.hCursor = LoadCursor16( 0, IDC_ARROW );
|
|
class16.lpszMenuName = (SEGPTR)0;
|
|
class16.lpszClassName = SEGPTR_GET(name);
|
|
for (i = 0; i < NB_BUILTIN_CLASSES16; i++, info16++)
|
|
{
|
|
class16.style = info16->style;
|
|
class16.lpfnWndProc = (WNDPROC16)MODULE_GetWndProcEntry16( info16->procName );
|
|
class16.cbWndExtra = info16->wndExtra;
|
|
class16.hbrBackground = info16->background;
|
|
strcpy( name, info16->className );
|
|
if (!RegisterClass16( &class16 )) return FALSE;
|
|
}
|
|
|
|
/* Create 32-bit classes */
|
|
|
|
for (i = 0; i < NB_BUILTIN_CLASSES32; i++, class32++)
|
|
{
|
|
/* Just to make sure the string is > 0x10000 */
|
|
strcpy( name, (char *)class32->lpszClassName );
|
|
class32->lpszClassName = name;
|
|
class32->hCursor = LoadCursor16( 0, IDC_ARROW );
|
|
if (!RegisterClass32A( class32 )) return FALSE;
|
|
}
|
|
|
|
SEGPTR_FREE(name);
|
|
return TRUE;
|
|
}
|
|
|
|
|
|
/***********************************************************************
|
|
* InitCommonControls (COMCTL32.15)
|
|
*/
|
|
void InitCommonControls(void)
|
|
{
|
|
int i;
|
|
char name[30];
|
|
WNDCLASS32A *class32 = WIDGETS_CommonControls32;
|
|
|
|
for (i = 0; i < NB_COMMON_CONTROLS32; i++, class32++)
|
|
{
|
|
/* Just to make sure the string is > 0x10000 */
|
|
strcpy( name, (char *)class32->lpszClassName );
|
|
class32->lpszClassName = name;
|
|
class32->hCursor = LoadCursor16( 0, IDC_ARROW );
|
|
RegisterClass32A( class32 );
|
|
}
|
|
}
|